Developer expertise is an easy idea of constructing the day-to-day of software program builders higher and simpler. I’m not going to elucidate it intimately as our CTO Marek Gajda already did a improbable job explaining why ought to we care about Developer Expertise in his textual content. On this article, I’ll go even deeper into technicalities and deal with how a programming language like TypeScript improves Developer Expertise.
JavaScript and Developer Expertise
JavaScript is a robust programming language, but you’ll be able to simply begin the journey with it. For example, each pc lately has an online browser with built-in developer instruments that help you experiment with the JavaScript engine straight away. What’s extra, the moment (seen) suggestions that you just get, might encourage you to go deeper.
There may be one drawback although, apps lately are extra complicated as customers demand from them greater than ever. JavaScript is not simply an decoration in your net web page; you construct fairly superior functions with it. This additionally implies that these apps can have tons of doable states, arduous to be absolutely lined by exams. And that’s the place JavaScript’s dynamic nature breaks the developer expertise as a result of prompt suggestions that the developer obtained is not the identical. Some runtime errors seem solely in particular app state mixtures.
Why does that occur? JavaScript is a really permissive language. As quickly because the JS code syntax is correct, it should run. Nonetheless, that form of “stress-free upbringing” received’t shield builders from writing code that fails on the runtime. What’s extra, the extra traces of code you have got, the larger the possibility that the code will set off runtime errors. In different phrases, once you have a look at the capabilities of the JS, there are extra methods to put in writing code that comprises runtime errors than the code that doesn’t.
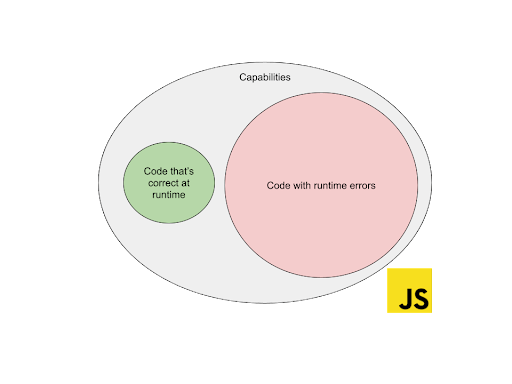
This clearly breaks the JS developer expertise in the long term – it’s simpler to create bugs than write code that offers with all the problematic circumstances gracefully.
The Elm method
Elm is a distinct segment programming language that’s domain-specific. Particularly, it targets the frontend area, the place JS has been the first choice for many years.
Elm implements the useful programming paradigm. Through the years builders found out that issues like immutability (a standard characteristic within the useful programming world) make our code extra maintainable in the long term.
Why even trouble with such a distinct segment programming language like Elm?
In the event you ask Elm programmers what’s their developer expertise in that language, most of them will guarantee you that it’s a lot better than it’s in JavaScript. So how does Elm obtain that? In the event you have a look at its capabilities, they’re much extra restricted:
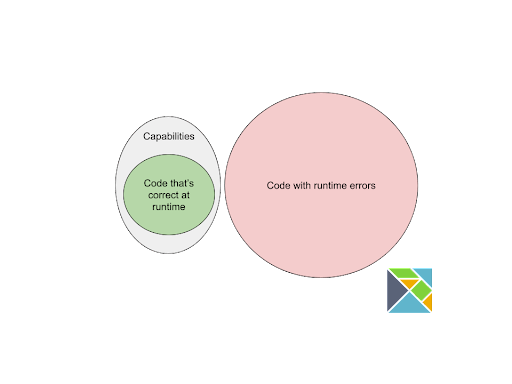
There’s a fairly common phrase throughout the Elm neighborhood: “if it compiles, it really works”.
Elm compiler doesn’t allow you to write code that fails at runtime. One of many options that make Elm stand out is its developer-friendly compiler suggestions.
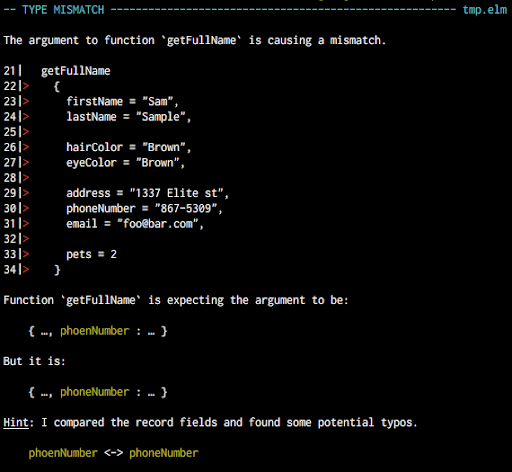
You possibly can most likely see that Elm compiler is NOT yelling on the developer that they did one thing mistaken. Elm permits you to determine this out for your self. As a substitute, it helps by offering as a lot context as it will possibly and hints at how the issue might be solved. In different phrases, the Elm compiler works as a dev’s assistant.
How does Elm obtain nice DX on a technical degree?
The useful programming paradigm is the one approach to write apps in Elm. But it surely’s value mentioning an extra profit – Elm can be a statically-typed programming language.
I wouldn’t like to begin a flamewar by way of what’s higher, dynamic or static typing. Each of those worlds have professionals and cons. Having mentioned that, I imagine that statically typed languages decelerate software program builders, for a superb purpose:
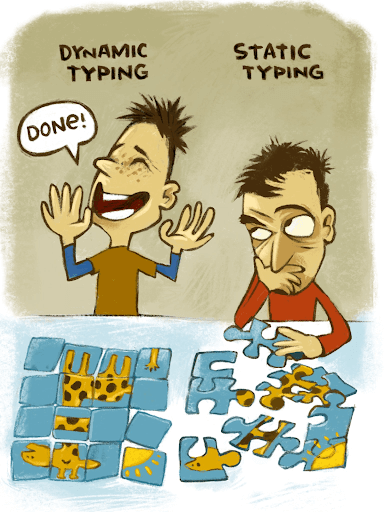
Why TypeScript?
What’s the cope with “Typescript improved Developer Expertise” then? In the event you check out the capabilities diagram once more, issues get fairly fascinating:
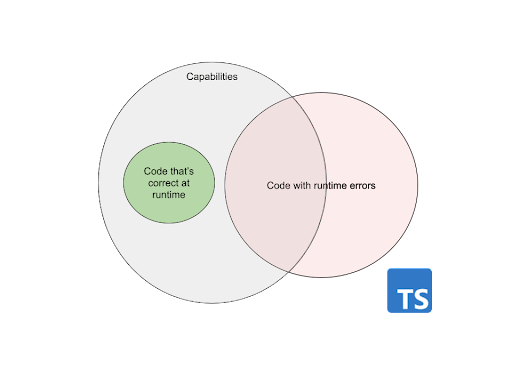
TypeScript is a programming language that could be a superset of JavaScript, so in fact, there’s nonetheless a chance to put in writing code that’s right on the runtime. Nonetheless, by way of the runtime errors, there’s some center floor between JS and Elm.
TypeScript permits you to stability between not-as-predictable dynamic JS world and statically typed code.
This can be a good compromise as a result of it creates a possibility to warn the developer if the compiler is for certain that one thing’s breaking at runtime. On the identical time, there’s at all times an escape hatch should you don’t need as a lot strictness for, let’s say, already present JS codebase that you just need to use with out additional effort. It is determined by you, the developer, how a lot time you’re keen to spend money on telling the TypeScript compiler concerning the code. In order a reward, you get some safety from the compiler that may inform you what might presumably go mistaken earlier than you even run the app.
In the event you look from the library and framework creators’ standpoint, good use of TypeScript options is a chance to create APIs that builders received’t hate. So on this article, I’m going to show TypeScript has the potential to take JavaScript to the heights of nice Developer Expertise through the use of some real-life examples and case research. So let’s dive into the code!
Case examine 1. The “common” perform
The issue
Take into account the next easy perform that calculates the common for an array of numbers:
It really works as anticipated for values like 5, 10, 15:
However, assuming that you’re an “common calculation area professional”, you realize about some edge circumstances:
Due to an empty array, you’ll divide zero by zero.
You possibly can simply think about that some React apps utilizing this library perform will get confused once they get rendered “NaN”.
Runtime error method
The very first thing that most likely pops into your thoughts is to repair this drawback. You should use an error throwing mechanism to speak the edge-case drawback to the developer:
Nonetheless, there’s an issue with this answer. TypeScript has no mechanisms that may power a developer to deal with native JavaScript errors. What’s extra, TypeScript doesn’t have native typed errors help in any respect. In the event you have a look at a “Promise” as an example, a profitable path might be simply typed, however the error path is typed as “any”. Which means that the aforementioned answer might make issues even worse.
Let’s pause for a second and consider using our perform with that React app context in thoughts. Wouldn’t it be a superb developer expertise to interrupt the app that programmers are engaged on by throwing an exception as a result of (in some part that summarises some knowledge from some desk) you miss numbers for the common perform?
One of many good developer expertise practices in libraries is “being a superb citizen”. Meaning your library code shouldn’t break the context the place it’s used. Throwing an error that crashes your React app is clearly a case that breaks this rule. So, we’ll have to check out various approaches.
Practical Programming vs Errors
Let’s contemplate how typical JavaScript capabilities behave:
Capabilities in JavaScript can:
- obtain and course of arguments,
- return output worth,
- carry out unwanted effects.
The final one, unwanted effects, is one thing that may simply spin your code uncontrolled. We had a superb instance of a aspect impact that may break the entire app within the earlier part, specifically, throwing an error is a aspect impact that may crash the entire app if the error shouldn’t be caught.
For comparability, let’s have a look at how a few of the useful programming languages cope with errors. In useful programming languages we have a tendency to make use of pure capabilities which are nearer to the mathematical definition of a perform:
Particularly, a pure perform can’t have unwanted effects. Like in math, you’ll be able to give it some thought as a black field that maps argument values to different values, that’s all. However the query arises: learn how to cope with the errors if there aren’t any unwanted effects?
If you implement pure capabilities there are solely two potentialities to speak an error. If the one approach to output one thing is the output worth, then why received’t you merely return the error as an output worth? If you revisit your code, you are able to do a easy, but highly effective change:
Did you discover how the “throw” key phrase has been changed with the “return” one?
Now, TypeScript is sensible sufficient to infer that perform might not return a numeric worth in all the circumstances. What’s extra, it will possibly warn the developer the place it’s doable to combine the numbers towards the error:
So, in such a case, builders are compelled to deal with the error the next means:
This trick nevertheless received’t shield you from the issue of getting one thing that’s clearly not a quantity rendered within the person interface. As a result of React received’t shield you from rendering “EmptyArrayError” so in that case, you’ll simply change “NaN” to “[Object object]”. No profit right here, maybe, that “NaN” alternative seems to be even worse on this case.
We have to attempt one thing else…
Narrowed sort method
Within the earlier part, you’ve seen that pure capabilities have solely two methods of speaking errors. We tried the “output worth” means, what’s left then? We have now no different choice than attempt from the “arguments” aspect.
In the event you don’t need to throw or return an error at runtime, outline your perform in a means that it’s unattainable to name with arguments that introduce the error. In math, you’ll be able to outline a site of a perform. It implies that some capabilities don’t have a way for values that don’t belong to the outlined area. TypeScript has a possibility to utilize that idea too. For instance, you’ll be able to outline a kind like this:
This works analogously to the built-in “Array<T>” sort. Nonetheless, the distinction is that it assures an array of a kind T having at the very least one factor. You should use such sort in your perform definition:
If you take a look at it, the completely happy path works like earlier than:
However, once you attempt the sting case:
…you’ll be able to clearly see that TypeScript protects you from writing code that doesn’t make sense:
There may be nonetheless one drawback although, in the true mission, these numbers will most likely come from the surface of your app (person enter, backend response, and many others.). So the compiler can’t foresee if the array that you just need to use shall be empty or not. However what you are able to do to deal with this drawback is to outline a constructor perform for our “NonEmptyArray” sort:
As you’ll be able to see, empty arrays must be dealt with by some means. In such circumstances, you’ll be able to resolve to return simply “undefined” however that’s only a matter of style what worth you need to return for that case.
Let’s simulate the state the place an array of numbers come from the world exterior of our TypeScript app:
Then, you may make use of the kind constructor perform as follows:
And what’s cool, it warns builders in the event that they need to do that:
This fashion, you’ll be able to information a developer that makes use of your library to implement the error dealing with as early because it might be:
At first look, this sample might look snippy. However in the long term, you shield the builders utilizing your library from inconvenient debugging classes on manufacturing, since you are sincere with them about what your library requires to have the ability to work accurately. With time, customers of your library will really feel self-confident that it received’t break their app as a result of they lined the edge-cases proper from the beginning, on their IDE degree.
Case examine 2. The “delay” perform
The issue
Let’s outline a easy perform making a promise that’s resolved with some delay:
Seems simple to make use of proper? Properly, for a programmer that is aware of JS by coronary heart it is perhaps fairly simple that such code will print “Hey” after 3 seconds:
However, in case you are an individual that spends tons of time writing some bash scripts the place the analogous “sleep” perform takes seconds as an argument, it’s possible you’ll make a mistake like this one:
It isn’t 100% clear what models the delay perform expects. We implement the developer to recollect what models they use and make room for ambiguities.
? It’s value remembering that unit ambiguity has brought on a couple of disaster up to now. Learn the article by my colleague beneath:
- What can net builders study from the costly errors of the area trade?
Nominal-types answer
To clear the ambiguities in terms of models, the nominal-type options come to the rescue. Nonetheless, in the meanwhile of writing this text TypeScript has no native help for this characteristic.
What’s nominal-typing? In our context, we will deal with it as a kind that we want to distinguish on a compiler degree, even whether it is related or equal to different sorts.
$100 ({dollars}) shouldn’t be the identical as 100€ (euros) though each are represented as a “quantity” equal to 100. By the best way, additionally it is value mentioning that the identical factor might be known as otherwise in different scopes, e.g. in stream these are opaque sorts.
So, TypeScript doesn’t help nominal sorts, proper? Properly, that’s solely partially true. As I’ve talked about, it doesn’t natively, however there are a few choices for simulating this characteristic. My favorite hack seems to be like this:
After introducing such sort, you’ll be able to outline sort constructors:
…and a few helper capabilities that make it doable to transform this sort again to a normal quantity sort:
Having these instruments in your toolbox means you’ll be able to rewrite the delay perform that it’ll not have an issue with time unit ambiguity:
So, the developer wanting to make use of it, can’t present only a quantity:
As a substitute, builders must be express concerning the time unit:
…and that’s cool since you present models which are rather more handy!
Worth object answer
The nominal-type answer jogs my memory of one thing that’s identified within the Area-Pushed Design as a Worth object. You possibly can implement a code that works just about the identical within the observe however is nearer to the Object-Oriented paradigm:
The one distinction is that in runtime you’ll go an actual object that wraps the numeric worth. In distinction, the nominal-type case has only a primitive quantity worth underneath the hood.
Case examine 3. “useQuery” React hook
The issue
One of many widespread issues in frontend apps is fetching knowledge from the backend. One approach to method that is to make use of hooks. So, let’s create a easy “useQuery” hook that may question the backend and deal with the request state.
INB4: I’m not protecting the runtime implementation itself so we will deal with the type-level of that code extra.
The standard states this hook ought to return embrace:
{ isLoading: true, success: false }
– when request has simply been despatched and also you watch for the response,{ isLoading: false, success: true, payload: { ... } }
– on profitable response,{ isLoading: false, success: false, error: NetworkError }
– community error.
Nonetheless, from the kind degree perspective, there’s nothing that stops the TypeScript compiler to deal with these absurd states as potentialities too:
{ isLoading: true, success: true }
– request in progress, however can be profitable, so what are we ready for?{ isLoading: false, success: true, payload: { ... }, error: NetworkError }
– we’ve bought success and error on the identical time. This jogs my memory of the well-known Shrödinger’s cat thought experiment.{ isLoading: true, success: true, payload: { ... }, error: NetworkError }
– loading + success + error combo.
All of those states match the ResponseState sort that we’ve outlined. In observe, should you write code that makes use of this hook:
Builders might not discover that this code received’t work accurately till they run the app. In observe, your hook wants a while to fetch the response, so the payload property that’s reserved for the profitable response received’t be obtainable on the primary part render. However, you’ve instructed the TypeScript compiler that payload is of sort “any” for all doable states, therefore TS compiler can’t assist the developer right here an excessive amount of.
Maybe there’s another approach to inform TypeScript which states actually do matter?
Tailored union sorts
Introducing, tailored union sorts! If you concentrate on significant request statuses in your case, begin with enum like:
Then, outline every of the doable response states as a separate sort. The best ones are for Idle and InProgress statuses:
Nonetheless, a profitable state is the place issues get fascinating:
You possibly can tie the payload property to be a singular property for the Success standing solely. Furthermore, you’ll be able to carry out analogously for the Error standing:
After defining all of those doable states one after the other, you’ll be able to mix all of them into one through the use of union sort characteristic:
So, once you use your hook with a typed return worth, TypeScript will warn builders that payload property might not exist:
Builders shall be compelled to show that they bought the right response state earlier than they’re able to get to the payload property:
This makes a pleasant alternative for code to be extra dependable as different (not profitable circumstances) must be explicitly dealt with (or devs shall be compelled to at the very least take into consideration them) earlier than the app compiles efficiently.
There may be nonetheless one situation although. Have you ever seen a bug within the aforementioned code? It is vitally delicate – a typo within the “remark.booy” half, it needs to be “remark.physique” as an alternative. I’ll cowl that within the subsequent part too.
Customization by way of generics
The issue with “any” sort is that it turns the TypeScript code again to a typeless JavaScript world, the place a variable might be something. In such a case, you would need to inform how the “payload” actually seems to be on a type-level as an alternative of the not-so-strict “any” sort. However you can not hardcode that by defining the stricter payload sort in your library degree as a result of your hook have to be extra generic and reusable for different backend endpoints that reply with totally different knowledge constructions.
Right here’s the place the generic sorts come to the rescue. Library authors typically method the issue of sorts customization by defining parameterizable generic sorts, so customers of their libraries can sneak their very own sorts in.
On this case, you can begin by making the profitable response generic:
Then, it must be propagated into locations that make use of that sort:
After doing this “generalization”, give the developer an choice to go their very own sorts like follows:
And, ta-da: TypeScript will catch typos now:
100% sort security
In the event you worth the type-safety even additional, you might also need to have a assure that backend responses match your frontend app assumptions. In different phrases, defining a kind like “Remark” received’t shield you from a backend that returns one thing utterly totally different. In order for you the developer to be 100% type-safe, you’ll be able to power them to go a response parser that ensures the information coming from the backend has the right construction:
In case one thing’s mistaken with the response knowledge, the responseParser perform can throw an error that may be caught and dealt with in your hook. For instance, you need to use zod library (which has a pleasant developer expertise by the best way) to outline the response parser to your feedback:
The highly effective mixture of generics and kind inference is the underestimated characteristic in TypeScript. If you go the outlined parser:
…you’ll be able to utterly omit the generic parameter, as TypeScript will work out the profitable response payload from the parser sort itself:
By the best way! Elm programming language offers with ensures vs backend communication compromise. Though the naming is a bit totally different as a result of as an alternative of calling it a parser, it calls it a JSON Decoder.
Developer expertise in TypeScript. Abstract
One of the crucial desired traits of an excellent developer expertise is nice documentation. However, what’s even higher is documentation that’s interactive and a design that guides the developer to the right utilization (of your API/library/code).
In distinction to overwhelming markdown books that train learn how to use your product, you are able to do one thing additional and supply builders with a mentor that may information them routinely by way of their use circumstances.
TypeScript is a robust device that may implement this method because of some good strategies that I’ve introduced on this article. I hope you have got been impressed to understand TypeScript as one thing greater than only a sort system for JavaScript, and that you’ll take pleasure in utilizing it as a device that may fill some developer expertise gaps.