In software program improvement, each coder has distinctive quirks and preferences that make their coding fashion distinct. However these differing approaches to naming conventions, indentation and spacing, error dealing with, and extra, could make crew collaboration difficult. That’s earlier than you even take into account unintuitive, advanced code. That is the place code feedback are available.
If you wish to know how one can write good feedback in code, it begins with them being significant. They need to clarify a developer’s reasoning and logic, appearing as a information that permits each crew member to grasp, preserve, and modify the code—regardless of after they be part of a mission.
On this publish, we’ll define the most effective practices and pitfalls to keep away from to advertise efficient data sharing and improve collaboration amongst builders.
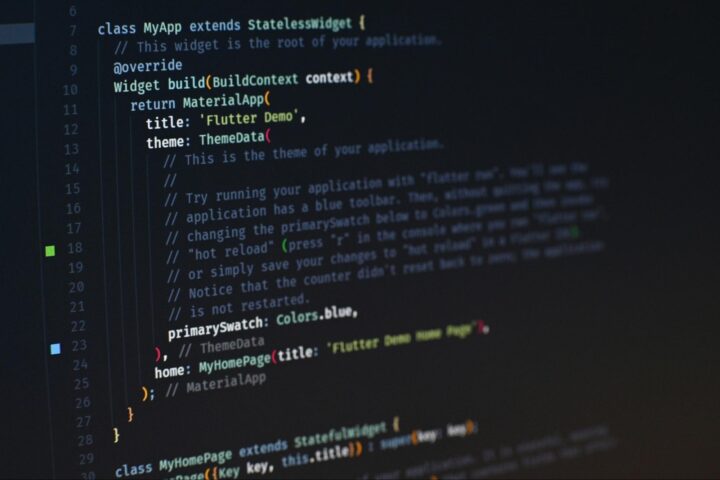
Code feedback are human-readable annotations builders embody inside sections of supply code. They add context to the code and make it simpler to grasp. Feedback are marked with particular characters to keep away from being evaluated by this system and, subsequently, don’t have an effect on the code’s habits.
Feedback ought to:
- Be written in pure language, utilizing accessible English statements.
- Clarify the code’s goal and performance.
- Describe any algorithms used to realize this.
- Spotlight different helpful implementation particulars.
- Flag up potential points or items of code that require explicit consideration.
Feedback sometimes use one in all two varieties of syntax based mostly on the size of supply code to be annotated.
The primary is known as a single-line/inline remark, which applies to a single line in this system. The second is a multi-line/block remark and describes a paragraph of textual content. Let’s take a look at every in additional element.
A single-line remark is positioned within the physique of the road of code. It describes and supplies a really temporary clarification of what’s happening. Use single-line feedback to assist crew members rise up to hurry with particular elements of code which are advanced or not intuitive.
Remember that commenting on each single line of code may have the precise reverse impact, rendering code much less readable.
Within the programming language Java, use // earlier than your remark for a single-line remark. This system will ignore all the things from // to the top of the road.
Block feedback present detailed explanations for a bit of code or advanced perform. They provide beneficial context, enabling crew members to grasp the general goal and logic of a code section to reinforce collaboration and guarantee smoother mission improvement.
In Java, use the next /* textual content */ for a block remark.
It’s necessary to keep in mind that you aren’t solely writing code to your pc to grasp and course of. It’s additionally a reference for your self and different crew members who you’re collaborating with.
Feedback make clear knowledge assortment strategies utilized in capabilities, aiding comprehension and teamwork. For instance, a remark like “This perform aggregates buyer knowledge from a number of sources utilizing our authorized knowledge assortment strategies, making certain consistency and reliability for advertising and marketing evaluation” provides clear insights into the information processes. That is useful for code upkeep, coaching new builders, and enhancing crew collaboration.
With extra corporations utilizing range recruiting platforms and trying to find distant expertise all over the world, there’s each probability that others in your crew have differing abilities and expertise.
Even when your code is well-written, there’s no assure that colleagues gained’t discover it complicated or ambiguous.
For broader mission insights, instruments like Microsoft Energy BI may be utilized to trace and visualize coding metrics or crew productiveness, which may be mentioned inside code feedback to additional improve understanding throughout the crew.
In relation to massive initiatives with distributed groups, code feedback additionally function a way of communication, serving to builders to work collectively seamlessly.
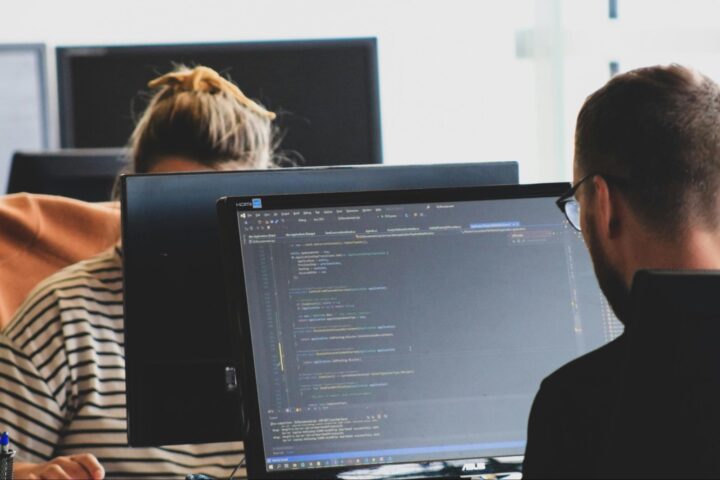
Let’s say a crew of builders is constructing a digital telephone system that permits customers to make and handle buyer calls over the web. One frontend developer would possibly work on coding for the system’s interactive dashboard whereas one other builds the visible name stream builder. A 3rd should create kinds for establishing auto-attendants and name routing guidelines constructed into the decision stream.
By participating with the code feedback left by the unique developer, the subsequent ought to have the ability to perceive and decide up on the prevailing implementation shortly and make the required adjustments with out interfering with the code’s performance.
We’ve established that offering efficient code feedback is vital to sustaining a readable, accessible, and maintainable codebase. Comply with these key greatest practices so you are able to do simply that whereas selling collaboration inside your improvement crew.
Give Extra Context
Use your remark to obviously clarify the why of your code—that’s, the context, logic, and reasoning behind it—slightly than merely what it does. This makes it simpler for future builders to grasp the intent of authentic builders, and means they’ll proceed to keep up, replace, or enhance the code with that in thoughts.
Let’s say you’re constructing an HR platform and your app depends on environment friendly knowledge retrieval to hold out high-volume hiring. As an alternative of offering an unclear code remark like “This perform codecs a date,” clarify the reasoning behind the code and why it’s necessary.
A remark like “This perform codecs a date to the ‘YYYY-MM-DD’ format to make sure consistency with the database storage necessities” instantly supplies builders with a extra readable, maintainable, and modifiable codebase to collaborate on.
Be Clear and Concise
Maintain feedback brief, targeted, and clear to keep up readability. Feedback shouldn’t state the plain however be restricted to essentially the most beneficial info.
Use easy, descriptive language, avoiding advanced terminology and jargon that might confuse crew members. Ensure your feedback don’t repeat the code itself or every other extraneous particulars that don’t add worth.
Right here’s an excessively wordy, advanced remark that accommodates pointless particulars:
“This perform, using a extremely optimized algorithm, iterates over the complete checklist of person objects to compute the overall variety of lively customers, making certain that the computational complexity stays linear, O(n). It begins by initializing a counter to zero, then it goes by way of every person within the checklist one after the other, checking if the person is lively, and if that’s the case, it increments the counter by one. Lastly, it returns the counter, which represents the overall variety of lively customers.”
A remark like this may undoubtedly trigger builders to make errors. They’ll battle to know the code’s goal and performance. What’s extra, they’ll possible spend vital time deciphering the remark, inflicting crew members to really feel alienated and hindering teamwork.
Right here’s a extra direct, concise various:
“This perform counts the variety of lively customers in a listing utilizing a linear scan to watch person engagement.”
This remark is extra accessible to crew members of all ranges, enhancing coding confidence and enhancing collaboration. It’s direct and clearly explains the perform and why it’s helpful with out overdoing it.
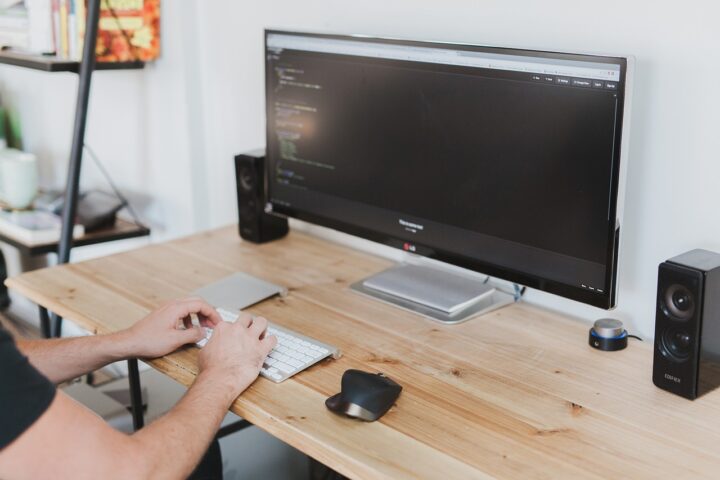
If you happen to moved from California to New York, you’d replace your quantity to a 646 space code. Equally, you must preserve and replace code feedback to replicate any adjustments in your codebase. It’s key to sustaining the accuracy and relevance of your feedback.
Outdated feedback trigger confusion. They’re open to misinterpretation and errors and compromise collaboration. In situations the place builders would possibly use voice-to-text instruments to shortly doc their code, making certain transcription accuracy is essential to keep away from miscommunications.
Transient your crew to additionally replace corresponding feedback after they modify code. Assessment feedback repeatedly and refactor them to weed out redundant ones.
Sustaining a constant commenting fashion all through your codebase boosts code readability and reduces misunderstandings.
To prioritize consistency, be sure to:
- Use the identical formatting strategy, corresponding to spacing and line size. This ensures feedback are straightforward to learn and builders can distinguish between feedback and code.
- Standardize using terminology throughout your codebase.
- Doc the circumstances/circumstances you’ve taken as true (assumptions) and the necessities you’ve met to your code to be executed efficiently (preconditions). This may assist builders grasp code context.
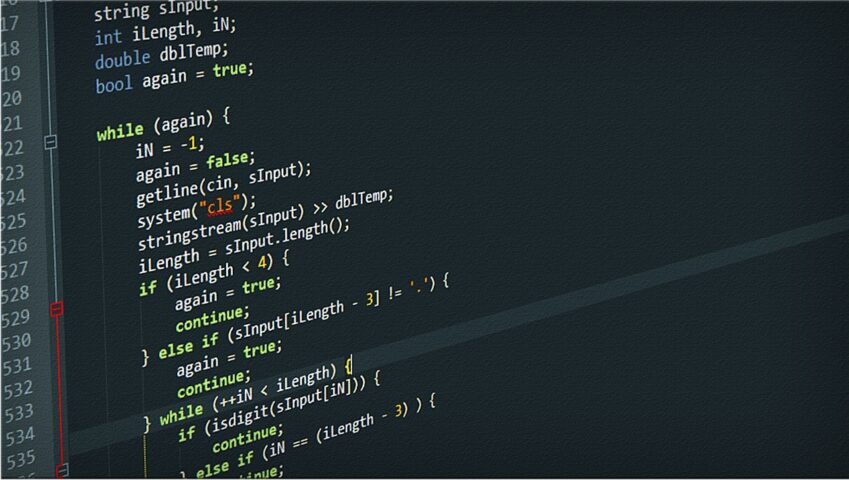
Make clear Advanced Code
Whether or not builders have simply completed their tech diploma or have been within the trade for years, non-obvious code may be obscure. Use feedback to make clear advanced logic and clarify why code is written the way in which it’s.
For instance, if you happen to’re coding a perform to calculate a reduction worth and be sure that the ultimate worth isn’t unfavourable, insert a remark explaining why this examine is critical so colleagues perceive the sting case it handles. Such a remark would possibly seem like this: “This handles circumstances the place the low cost is larger than the worth”.
Equally, when coping with area identify validation or routing logic in an online software, clear feedback may help different builders perceive how domains are processed and keep away from potential misconfigurations.
Widespread Pitfalls to Keep away from for Higher Collaboration
We’ve established how one can write significant feedback that foster collaboration. However let’s take a look at essentially the most frequent errors builders make that usually find yourself alienating crew members and inflicting errors.
Over-Commenting and Underneath-Commenting
Putting the appropriate stability with the variety of feedback you embody inside your code is vital. Over-commenting creates muddle, which obscures logic and goal, is difficult to learn and preserve, and stymies collaboration.
Then again, under-commenting leaves vital gaps in understanding, particularly for much less skilled builders and new crew members.
Present sufficient info to clarify the context and performance of code, notably advanced or non-obvious sections, with out overwhelming the reader.
rule of thumb is to make no multiple remark per ten traces of code.
Feedback shouldn’t be used as a crutch to clarify complicated or overly advanced code. Counting on feedback to clarify unhealthy code makes the codebase more durable to grasp and preserve in the long term.
Prioritize writing clear, readable code first, then use feedback to reinforce readability the place obligatory.
Whether or not you’re embarking in your first 100 days of code or are a seasoned developer, understanding the worth of writing clear and informative feedback is crucial for individuals who try to keep up a readable and modifiable codebase.
Good feedback are clear, up-to-date, constant, and useful. They permit builders to grasp one another’s work and contribute to initiatives effortlessly. Because of this, all crew members really feel empowered to work collectively in producing greater code high quality and, in the end, extra profitable mission improvement.
Land Your Dream Entrance Finish Net Developer Job in 2024!
Be taught to code with Treehouse Techdegree’s curated curriculum stuffed with real-world initiatives and alongside unbelievable pupil help. Construct your portfolio. Get licensed. Land your dream job in tech. Join a free, 7-day trial in the present day!
Begin a Free Trial
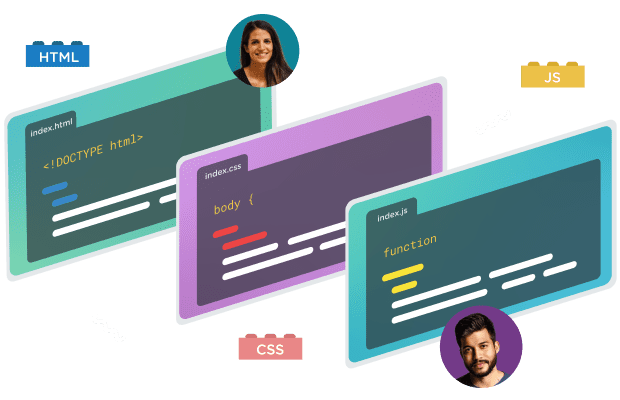