Issues on the web are all the time altering. Generally web sites themselves change. Generally issues on a web site dynamically change values whilst you’re interacting with it. That is the place my curiosity peaks.
I’ve all the time observed little issues like this in life and puzzled the way it’s occurring behind the scenes. I can stroll as much as a merchandising machine, put in no matter loopy amount of cash they’re charging lately, select, and the machine delivers my desired snack. . .if their {hardware} is working, after all.
I do know that there’s not a bit of merchandising machine gremlin hiding inside, operating round exhausted, pushing choices out, counting change, and many others. (desires shattered). So my mind begins considering of what parts are linked, what’s the chain of occasions from the second I insert my cash earlier than I’m devouring my spicy chips or doughnuts?
Turn into a Full Stack JavaScript Developer Job in 2024!
Be taught to code with Treehouse Techdegree’s curated curriculum stuffed with real-world initiatives and alongside unimaginable pupil help. Construct your portfolio. Get licensed. Land your dream job in tech. Join a free, 7-day trial as we speak!
Begin a Free Trial
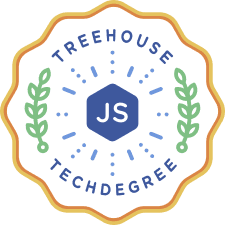
Fortunately for us, in JavaScript, that is very simple to do. We are able to dynamically change the worth of 1 component based mostly on one other and we are able to accomplish that in real-time. This turns into particularly useful when working with kinds.
Now that I’ve doughnuts on my thoughts, let’s follow this merchandising machine concept and simplify it to an HTML kind for this instance. Let’s think about there are two machines aspect by aspect: one containing drinks and one containing snacks.
Setting the Stage with HTML
Right here’s my primary HTML. I’ve a kind component that accommodates a choose component on the high. That is the place we determine which machine to strategy, drinks or snacks. Beneath that’s one other choose component with all our drinks and snack choices. I’ve utilized a knowledge attribute to those to distinguish them inside our JavaScript later. Lastly, beneath that, we’ve got a submit button.
<kind>
<choose title="machineSelect" id="machineSelect">
<possibility worth="default" chosen hidden disabled>
Choose a Machine
</possibility>
<possibility worth="drinks">Drinks</possibility>
<possibility worth="snacks">Snacks</possibility>
</choose>
<choose title="itemSelect" id="itemSelect">
<possibility worth="default" chosen hidden disabled>Choose an Merchandise</possibility>
<possibility data-category="drinks" worth="water">Water</possibility>
<possibility data-category="drinks" worth="soda">Soda</possibility>
<possibility data-category="snacks" worth="chips">Chips</possibility>
<possibility data-category="snacks" worth="donuts">Donuts</possibility>
</choose>
<button sort="submit">Buy</button>
</kind>
Bringing the Kind to Life with JavaScript
So, what’s our aim right here? We wish to, in real-time, solely present related objects in our second choose menu based mostly on what the shopper has chosen within the first. It wouldn’t make sense in our case to even have two totally different machines in the event that they each bought drinks and snacks, regardless of how a lot gremlin labor that might save.
First issues first, in our JavaScript, we want retailer reference to a couple key parts that we are able to entry over time: every of our choose parts and every of the merchandise choices throughout the second menu. I’ll retailer the primary two in variables utilizing the querySelector methodology. Discover for the choices, I’m utilizing querySelectorAll, grabbing the choices straight from the itemSelect component and provided that they’ve that data-category attribute. It is because I’m storing multiple component, I solely need choices nested inside that particular component, and I wish to keep away from getting reference to the primary default possibility. I’d additionally prefer to disable the itemSelect menu upon loading the web page. This might be our little method of stopping the wandering toddler from hitting buttons and getting free snacks.
// retailer our obligatory parts in variables
const machineSelect = doc.querySelector("#machineSelect");
const itemSelect = doc.querySelector("#itemSelect");
const itemOptions = itemSelect.querySelectorAll("possibility[data-category]");
// disable the merchandise menu upon web page load
itemSelect.disabled = true;
Alright, so within the occasion of adjustments occurring to the machineSelect, we have to add a technique to pay attention for that change. That is very simple by utilizing JavaScript’s addEventListener methodology and specifying the “change” occasion… see what I did there? I’ll see myself out shortly.
We are able to then go it an nameless perform utilizing the arrow syntax and add our code inside.
// pay attention for adjustments on the machine menu
machineSelect.addEventListener("change", () => {
// code we wish to run on each change
});
The very first thing we should always do is allow our itemSelect component to allow them to work together with it now. The proud gremlin grants permission for the consumer to pick out an merchandise.
// allow the merchandise menu
itemSelect.disabled = false;
Dynamically Change Worth Based mostly on Person’s Alternative
Now we’ve hit our problem. At present, all our merchandise choices seem when opening the second menu, even when they don’t correlate to the kind of machine chosen, permitting room for consumer errors in our kind.
We have to loop over our itemOptions, examine what its data-category worth is, and conceal the choices that don’t match up. We are able to use just a few several types of loops right here, however I want a forEach loop since I do know I’m going to should iterate over each merchandise each time, and I want the straightforward syntax.
// loop over every merchandise possibility
itemOptions.forEach((merchandise) => {
}
The very first thing I would like is the at the moment iterated-over merchandise’s data-category worth. We are able to use the getAttribute methodology to perform this and retailer the returned worth right into a variable.
itemOptions.forEach((merchandise) => {
// retailer the merchandise's class worth
const class = merchandise.getAttribute("data-category");
}
Should you look again at our HTML, you’ll discover I’ve purposefully given the data-category’s values the identical strings because the values on our machineSelect’s choices. This makes the following step handy for me, however for those who don’t have this sort of management over what you’re engaged on, you could have to take an additional step in evaluating these values.
Now looks as if a good time for a conditional assertion. With this setup, we are able to merely examine if our new variable shouldn’t be strictly equal to the machineSelect’s worth. If this situation is true, that means they don’t match up, we merely conceal this present possibility. I’ve additionally added the disabled attribute as a result of some browsers like being totally different and troublesome for us devs *ahem* Safari *cough sound*. If the consumer is indecisive whether or not they’re hungry or thirsty and make a number of adjustments to the machineSelect, we have to be sure that beforehand hidden and disabled choices are seen and enabled once more within the else block.
// conceal non-matching choices, show matching ones
if (class !== machineSelect.worth) {
merchandise.hidden = true;
merchandise.disabled = true;
} else {
merchandise.hidden = false;
merchandise.disabled = false;
}
Superior! Solely our related objects seem as choices within the second choose menu!
Turn into a Full Stack JavaScript Developer Job in 2024!
Be taught to code with Treehouse Techdegree’s curated curriculum stuffed with real-world initiatives and alongside unimaginable pupil help. Construct your portfolio. Get licensed. Land your dream job in tech. Join a free, 7-day trial as we speak!
Begin a Free Trial
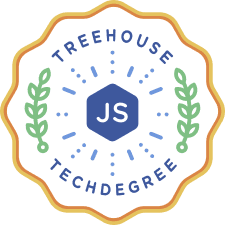
However beware! There may be nonetheless a chance of a really sneaky difficulty that may trigger a consumer error right here. If, for instance, I select the “Drinks” machine after which choose “Soda” as an merchandise, then my abdomen growls and I understand I solely manage to pay for for one merchandise and I ought to most likely get some doughnuts as a substitute, and change to the “Snacks” machine, our objects menu nonetheless has our “Soda” choice. This clearly couldn’t occur in actual life after all, however this leaves room for me to submit this manner with a mismatched choice. This may occasionally confuse and anger our gremlin pal.
Resetting Selections for Consistency
It might be superb if each time the consumer adjustments the machine, the itemSelect menu both: adjustments again to the default possibility, or we assign an identical possibility as a default. I’ll go along with the primary possibility so it’s apparent they should make a brand new choice and don’t accidently submit with an possibility I supplied.
So, after our loop, we are able to merely assign our itemSelect’s worth to the worth of our default possibility, which for those who look again on the HTML, I’ve made it the string “default”.
// reset the worth of the merchandise menu
itemSelect.worth = "default";
Now, every time the machine adjustments, our merchandise menu is introduced again to the default chosen possibility. Good!
Right here’s the JavaScript in entire.
// retailer our obligatory parts in variables
const machineSelect = doc.querySelector("#machineSelect");
const itemSelect = doc.querySelector("#itemSelect");
const itemOptions = itemSelect.querySelectorAll("possibility[data-category]");
// disable the merchandise menu upon web page load
itemSelect.disabled = true;
// pay attention for adjustments on the machine menu
machineSelect.addEventListener("change", () => {
// allow the merchandise menu
itemSelect.disabled = false;
// loop over every merchandise possibility
itemOptions.forEach((merchandise) => {
// retailer the merchandise's class worth
const class = merchandise.getAttribute("data-category");
// conceal non-matching choices, show matching ones
if (class !== machineSelect.worth) {
merchandise.hidden = true;
merchandise.disabled = true;
} else {
merchandise.hidden = false;
merchandise.disabled = false;
}
});
// reset the worth of the merchandise menu
itemSelect.worth = "default";
});
I’ll go away it at this, however I encourage you to get some observe in and increase on this additional! You may add an eventListener on the shape itself and pay attention for the “submit” occasion and let the consumer know you’re sorry, however the gremlin ate your snack already, however thanks for the cash. Or you’ll be able to implement some validation, making certain the consumer has made an merchandise choice.
You can add in one other choose menu or enter discipline to see how a lot cash the consumer is inserting, apply a value to every merchandise and calculate the change that the gremlin will most likely pocket anyway.
The machineSelect may be radio buttons as a substitute of a choose component. The sky’s the restrict in terms of snacks… I imply JavaScript!
This additionally doesn’t should be applied solely in kinds, after all. You may pay attention for all types of occasions on all types of parts, get values, set different values, and convey your webpages to life! I hope this proves useful for any person, I’m off to search out some doughnuts. Glad coding everybody!
Increase Your Coding Expertise: Begin Your Free 7-Day Trial
Able to take your coding abilities to the following degree? Enroll now for a free 7-day trial and unlock limitless entry to our in depth library of coding sources, tutorials, and initiatives. Whether or not you’re a newbie or an skilled developer, there’s all the time one thing new to study.
Don’t miss out on this chance to increase your data and develop your coding experience. Be a part of us as we speak and begin your journey in the direction of mastering coding!