TypeScript grew to become an ordinary for backend (Node.js) and frontend (Angular/React) growth. The categories it brings enable us to develop apps with the boldness that we gained’t make any foolish errors. It doesn’t relieve us from the accountability of utilizing finest coding practices, but it surely does make builders’ lives a bit simpler. But there may be one draw back of TS. It’s compile time. So, let’s speak about learn how to pace up your TypeScript undertaking.
Optimizing TypeScript tasks is one thing we pay a number of consideration to. Not solely is it one among our key applied sciences, the State of Frontend 2022 reveals that that is how nearly all of frontend builders really feel today – 84,1% of all respondents admitted to utilizing TypeScript over the past yr!
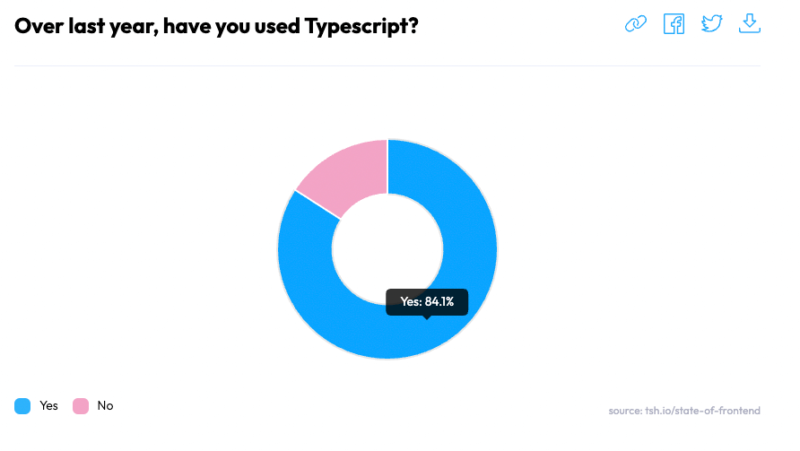
So, how are you going to enhance the pace of your TypeScript undertaking?
What you’ll be taught
There are numerous approaches, however on this one I’m going to give attention to shortening the transpilation time to JavaScript. Aside from that, you’re going to seek out out about:
- utilizing the Superfast JavaScript/TypeScript Compiler (SWC),
- varied methods to make use of the TypeScript compiler, together with the – –noEmit flag,
- our personal Specific Boilerplate and the way in which we use at The Software program Home it to optimize tasks.
Let’s begin with some primary information about compiling TypeScript.
Compiling TypeScript – the woes of tsc use
The extra code you have got, the longer it takes to compile. I feel we are able to all agree on that. In some circumstances, that’s additionally the rationale why we cut up the applying into smaller items (companies), so we don’t have to compile all the undertaking and file system, together with json file and all the remainder.
Let’s take our Specific Boilerplate (the starter we’re utilizing to construct apps for our shoppers). To hurry up the event course of, no matter what number of recordsdata the undertaking references, we’ve constructed a starter pack that displays our way-to-go stack.
This contains:
- production-ready containerization,
- TypeScript assist,
- Plop for code technology,
- built-in assist for Redis and PostgreSQL with TypeORM,
- finest practices like Command sample or Dependency Injection with Awilix
- REST/graphQL API assist,
and extra!
In its uncooked kind, working the `npm run watch` command (which begins the TypeScript Compiler – tsc – in a watch mode) takes 28 seconds to have an atmosphere able to work with.
It doesn’t sound like rather a lot, however with extra code to return, this quantity will slowly improve. So what occurs throughout TypeScript compilation?
TypeScript compilation course of
In brief, there are two main elements:
- sorts checking,
- transpilation to JavaScript
At this level we can’t do a lot with sort checking, nonetheless, there’s a strategy to pace up the compilation pace.
TypeScript itself can’t change TS supply code into JS recordsdata. There isn’t any such performance. Nevertheless, there may be one that permits us to test sorts solely – –noEmit flag.
So what if we used one thing quicker for a transpilation half and use TSC just for TS sorts checking?
Introducing… SWC!
Bettering the effectivity of TypeScript-based tasks is what we do.
Take a look at the case examine of a serious occasions platform to see how we enhanced the scalability and value of the AWS- and TypeScript-based software.
What’s SWC?
SWC stands for Superfast JavaScript/TypeScript Compiler. It’s a software, created in Rust, that has one job – to transpile the code from TS/ES to a particular goal. It gained’t test the categories (at the very least not but), however due to that, it’s a lot quicker than TSC.
Our Boilerplate compiles in 7 seconds. That’s 4 instances quicker than utilizing the built-in TypeScript instruments. So how does it work?
SWC will be put in as normal devDependency with:npm i -D @swc/core @swc/cli
And that’s all! Not less than for some primary options. ?
You possibly can strive it by working:
swc <some-directory> --out-dir construct
… and in a second you need to get the code transpiled. If it’s essential to have a watch mode, then simply add the -w flag on the finish.
In fact, it’s a little bit extra difficult for extra complicated tasks. By default SWC has a lot of the options turned off and for a lot of TypeScript purposes, it is going to be needed so as to add further configuration
This might be performed with a particular single file: .swcrc file. Take a look at the next code:
Sadly there is no such thing as a software that creates swc configuration from tsconfig but. Due to this fact, you need to keep in mind to maintain each recordsdata in sync.
There are a couple of helpful keys within the SWC config.
The primary one is jsc. This key incorporates every part associated to the parser sort we’re going to make use of – for instance typescript or ECMAScript, but additionally some details about the goal js model we would like our code to be transformed to.
The second is a module. This one is very helpful for Node.js apps, due to the CommonJS modules requirement.
For many of our apps, we additionally needed to activate the decorators assist, in any other case you’ll get a nasty error message.
Clearly, these are just a few of the various potential configuration choices. Be at liberty to test the remaining ones on the SWC configuration web site.
What about sorts?
One query stays unanswered. Will we drop sorts checking? In reality, SWC doesn’t care concerning the code validity nor it cares about sorts. So in actuality we’re dropping the largest motive to make use of TypeScript for. What the hell?! Don’t fear we aren’t doing that. ?
In the beginning of this text, I discussed that TS can work in two methods. It both generates the code and checks sorts or solely checks sorts. Let’s use the second method then!
The command we want is easy:
tsc -w --pretty --skipLibCheck --noEmit
But we nonetheless have to run each in parallel – SWC and TSC. We are able to both use a library for that (to have cross-operating system performance) or we are able to simply use and run each concurrently.swc src --out-dir construct/src -w --sync & tsc -w --pretty --skipLibCheck --noEmit
What’s the good thing about that method?
The app is able to develop in 7s, and on the identical time, we’re going to get all the details about potential errors in our code.
The bigger our app will get, the larger the distinction between SWC and TSC goes to be, so having the likelihood to develop earlier is a large benefit.
In fact, there are different subjects associated to the –noEmit flag, which I’ll not get into this time. Please, take a look at and be taught extra about: declaration recordsdata, the bundle dependency graph, or the tsbuildinfo file.
So… learn how to pace up your TypeScript undertaking?
So the place is the catch? – it’s possible you’ll ask. Nicely, there are a few issues to recollect:
- The TypeScript code generated with SWC is a bit bit totally different from the one generated by TSC. In some circumstances, this leads us to some points with our tsc-working software. For instance, relying on a configuration, embellished lessons are returned as lessons or objects (but in tsc it’s at all times going to be a category).
- It doesn’t sound dangerous, however if any of your features requires the precise sort (for instance class), it’ll break. We had this downside with the Awilix helper asClass.The opposite downside is the assist of recent options.
- SWC is evolving fairly shortly, but it nonetheless doesn’t assist all the options that TSC/Babel does. Essentially the most up-to-date checklist of options is accessible on the SWC web site. However it is usually price checking the GitHub points pages.
So would I like to recommend utilizing SWC?
There’s a shiny facet right here. It is rather simple to alter TypeScript code and swap backwards and forwards between SWC and TSC, so I’d say all of us ought to give it a strive!
At The Software program Home we’re going to play with this a bit bit extra to see how steady it’s in bigger tasks. Nevertheless, at this level I’m very proud of the outcomes!
Do you need to construct quicker with TypeScript? This is only one of many questions it’s essential to reply in your subsequent undertaking.
Contact The Software program Home and sort out all of them. Preliminary consultations are freed from cost!