Dependency injections, when applied correctly, provide plenty of advantages. They will make your system extra versatile and modular, and even aid you write testable code. Sadly, the implementation is just not simple and it’s particularly hardly ever used with regards to Node.js. However at this time I’m going to indicate you that not solely is dependency injection in Node.js a factor, but additionally that it may be achieved with features too! Let’s get to coding!
Whenever you begin working with Node.js, one of many first ideas you be taught is the module sample. There may be nothing higher than reusability and modularity, isn’t it?
However in accordance with some, you don’t want dependency injection in Node.js as a result of there’s require. Personally, I don’t actually agree with that. Why?
Let’s begin by clearly establishing what JavaScript dependency injection in Node.js really is.
What you’ll be taught – the dependency injection in Node.js overview
In at this time’s article, I’m going to indicate you the potential of dependency injections in Node.js to make your life simpler. Particularly, you’re going to get:
- sensible rationalization of the dependency injection idea (We’re going to jot down code examples),
- an outline of dependency injection options in Node.js for each object oriented programming and features (sure!),
- a fast introduction into establishing a DI-based mission, specifically the orchestration and tooling.
Let’s go for it!
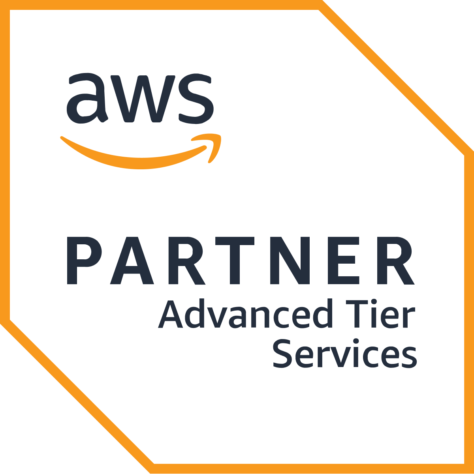
What’s a dependency injection in Node.js?
Dependency injection in Node.js or JavaScript generally is a widely known method, which may make it a lot simpler to provide impartial and scalable modules. It may be utilized in many programming languages and frameworks. Nonetheless, with regards to Node.js, dependency injections aren’t fairly as in style as they might be. To some extent, it’s a results of sure misconceptions.
To place it in different phrases, dependency injection in Node.js is a sample the place, as a substitute of making or requiring dependencies straight inside a module, we go them as parameters or reference.
At first look, it could be fairly obscure. However it’s simpler than you suppose.
Let’s think about a easy service module:
It appears superb. The service is answerable for enterprise logic and person repository is answerable for communication with the information supply. However there are two issues right here.
Initially, the service is coupled with a particular repository. If we wished to vary it to one thing else, then we must modify your complete code. I’m positive you realize one of many widespread programming guidelines – program to an interface, not an implementation. That is the place we violate that rule!
A second drawback is the testability of this module. If we wished to seek out out if getUsers
technique works, we would want to stub usersRepository
utilizing Sinon, Jest.mock or every other stubbing library.
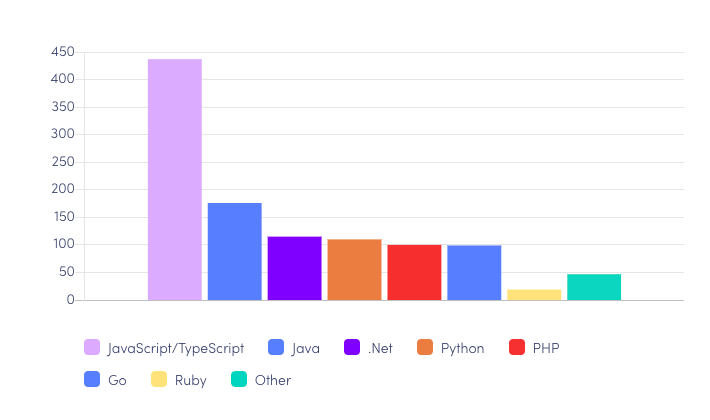
Want top-class Node.js growth?
? Depend on Poland’s greatest Node group to construct or develop your app with success. Expertise professionals fee our software program growth supply 4.9 on Clutch.
It appears sophisticated, doesn’t it? Let’s use dependency injection to repair it up!
What we have to do is to go usersRepository
as a parameter as a substitute of requiring it straight.
As you possibly can see, the service is not paired with a repository module however requires usersRepository
to be handed to it. It has a serious affect on testability:
We might drop sinon from our dependencies and change it with the injection of the customers repository. This method permits us to unit-test far more advanced instances, with out the necessity for stubbing.
What’s extra, by decoupling service and repository, you achieve the liberty to vary implementation at any level. Cool!
Dependency injection in Node.js – lessons vs features
One more reason why dependency injections aren’t in style within the Node.js ecosystem could be a fantasy that DI is an OOP-only idea. That’s most positively not true!
After all, it’s apparent how one can inject dependencies in lessons. Now we have a constructor, the place we will inject dependencies one after the other or by a single object. At TSH, we’re large followers of decreasing the variety of params being handed to class/perform by enclosing them within the object.
You may simply use destructuring to entry particular dependencies you want. It’s even higher in TypeScript the place you possibly can specify required dependencies by sort.
However JavaScript is just not solely about lessons (everyone knows that it’s simply syntax sugar). So how can we obtain the identical with features solely? The reply is easy – parameters.
We create a perform that takes dependencies as parameters after which returns one other perform/object with a particular implementation. Due to the closure we created, we have now entry to dependencies from the inside features.
As you possibly can see, DI is just not solely about lessons!
Orchestration and tooling
The seen draw back of DI is the requirement to set every little thing up beforehand. Following the earlier instance, if I need to create customers service, I have to make a repository, mailer and logger. What’s extra, each repository and mailer might have their very own dependencies, so I have to create the entire construction. Let’s make a dependency injection container. We’re additionally going to create some configuration information.
We have to put together some issues earlier than we’re capable of create the service. This method is what we name service container – a particular module the place the entire public providers/factories and so forth are orchestrated.
After all, this one is easy and requires plenty of guide work. Nonetheless, there are Node.js DI libraries that may do a few of it for us.
There are just a few choices out there. The preferred ones are Awilix, Inversify and TypeDI.
TypeDI and Awilix are fairly related and work with each JavaScript and TypeScript. However, Inversify is TypeScript-only.
At TSH, we’re principally utilizing Awilix, however think about TypeDI to be very promising.
By utilizing Awilix, we will create a particular container that may resolve dependencies by itself. The one factor we have to present is base constructing blocks. In our case, it’s about providers like DataSource, Logger or parameters like stage
or templates
.
The remaining (UsersService, UsersRepository, Mailer) can be resolved mechanically by Awilix. The one factor we have to present is a sort of resolver (for instance, we have to inform that UsersService is a category).
After I name the resolve
technique on an Awilix container, it goes via the entire constructor/perform parameters and checks if there’s a dependency with the identical identify in our container (Awilix answer is just not based mostly on varieties – even for TS – however on the dependency identify) and tries to resolve it.
By doing this, we don’t have to create our dependencies one after the other. We solely want to supply constructing blocks after which name the resolve technique to get a particular service.
I strongly encourage you to verify each Awilix and TypeDI (for TypeScript, it permits to get dependencies by varieties).
Options for dependency injection in Node.js – pals or foes?
That’s about it! Let’s sum issues up:
- Node dependency injection supplies plenty of flexibility not solely with regards to simpler unit testing and testing generally, but additionally when working with an app.
- It permits us to create and implement modules which can be totally impartial of one another and it’s at all times a welcome factor in trendy object oriented programming and software program growth. Most builders can respect the advantages dependency injection sample supplies.
- By utilizing some further instruments, for instance a dependency container, you may make the duty of modules constructing ever simpler, avoiding fairly just a few points introduced up on this article.
At TSH we use DI in each Node.js mission, in each small and enormous tasks, and we will’t think about working with our code with out it.
I’m going to return to the subject of dependency injection in Node.js in addition to nicely as different design patterns reminiscent of dependency inversion and dependency inversion precept within the coming articles so keep tuned!
Are you in search of Node.js builders who can use dependency injection in business tasks?
Our portfolio is stuffed with sensible implementations of comparable strategies and ideas. Test it out!