Frameworks like Laravel and Ruby on Rails get rid of numerous choices that you’d in any other case be pressured to make when constructing internet purposes. Even so, a substantial amount of time is spent on mundane duties fairly than constructing the mental property that makes the appliance distinctive. One such job includes producing database seed recordsdata. On this submit I’ll present you the way you should utilize ChatGPT to generate each static and dynamic database seed recordsdata and rapidly populate your improvement database with life like information.
What’s a Database Seed File?
When constructing an internet utility it is vitally helpful to in style your person interfaces with realistic-looking information. A few of this information is faked, which means for example randomly generated person names and electronic mail addresses, whereas different information is templated, which means it consists of static lists corresponding to US states and Main League Baseball groups.
As soon as this information has been imported into your improvement database, it makes constructing out the assorted pages and dashboards way more life like. For instance right here is an web page populated with faux customers. This check information is imported into the event database utilizing a seed file. As an illustration a Laravel-based seed file containing US states may seem like this:
<?php
namespace DatabaseSeeders;
use IlluminateDatabaseConsoleSeedsWithoutModelEvents;
use IlluminateDatabaseSeeder;
use AppModelsState;
class StateSeeder extends Seeder
{
/**
* Run the database seeds.
*/
public operate run(): void
{
State::truncate();
$states = [
["name" => "Alabama", "abbreviation" => "AL"],
["name" => "Alaska", "abbreviation" => "AK"],
["name" => "Arizona", "abbreviation" => "AZ"],
["name" => "Arkansas", "abbreviation" => "AR"],
["name" => "California", "abbreviation" => "CA"],
["name" => "Colorado", "abbreviation" => "CO"],
["name" => "Connecticut", "abbreviation" => "CT"],
["name" => "Delaware", "abbreviation" => "DE"],
["name" => "District of Columbia", "abbreviation" => "DC"],
["name" => "Florida", "abbreviation" => "FL"],
["name" => "Georgia", "abbreviation" => "GA"],
["name" => "Hawaii", "abbreviation" => "HI"],
["name" => "Idaho", "abbreviation" => "ID"],
["name" => "Illinois", "abbreviation" => "IL"],
["name" => "Indiana", "abbreviation" => "IN"],
["name" => "Iowa", "abbreviation" => "IA"],
["name" => "Kansas", "abbreviation" => "KS"],
["name" => "Kentucky", "abbreviation" => "KY"],
["name" => "Louisiana", "abbreviation" => "LA"],
["name" => "Maine", "abbreviation" => "ME"],
["name" => "Maryland", "abbreviation" => "MD"],
["name" => "Massachusetts", "abbreviation" => "MA"],
["name" => "Michigan", "abbreviation" => "MI"],
["name" => "Minnesota", "abbreviation" => "MN"],
["name" => "Mississippi", "abbreviation" => "MS"],
["name" => "Missouri", "abbreviation" => "MO"],
["name" => "Montana", "abbreviation" => "MT"],
["name" => "Nebraska", "abbreviation" => "NE"],
["name" => "Nevada", "abbreviation" => "NV"],
["name" => "New Hampshire", "abbreviation" => "NH"],
["name" => "New Jersey", "abbreviation" => "NJ"],
["name" => "New Mexico", "abbreviation" => "NM"],
["name" => "New York", "abbreviation" => "NY"],
["name" => "North Carolina", "abbreviation" => "NC"],
["name" => "North Dakota", "abbreviation" => "ND"],
["name" => "Ohio", "abbreviation" => "OH"],
["name" => "Oklahoma", "abbreviation" => "OK"],
["name" => "Oregon", "abbreviation" => "OR"],
["name" => "Pennsylvania", "abbreviation" => "PA"],
["name" => "Rhode Island", "abbreviation" => "RI"],
["name" => "South Carolina", "abbreviation" => "SC"],
["name" => "South Dakota", "abbreviation" => "SD"],
["name" => "Tennessee", "abbreviation" => "TN"],
["name" => "Texas", "abbreviation" => "TX"],
["name" => "Utah", "abbreviation" => "UT"],
["name" => "Vermont", "abbreviation" => "VT"],
["name" => "Virginia", "abbreviation" => "VA"],
["name" => "Washington", "abbreviation" => "WA"],
["name" => "West Virginia", "abbreviation" => "WV"],
["name" => "Wisconsin", "abbreviation" => "WI"],
["name" => "Wyoming", "abbreviation" => "WY"]
];
DB::desk('states')->insert($states);
}
}
Even for those who don’t know something about Laravel, the code ought to be pretty comprehensible. I created a multidimensional array consisting of every state identify and its corresponding two character abbreviation.
Previous to instruments like ChatGPT and Copilot, for those who needed to create a seed file containing US States, you’d sometimes go to Google, seek for one thing like “US State names and abbreviations”, after which painstakingly copy and paste the end result into an array. Over time, builders understandably grew to become bored with this kind of work and created weblog posts and GitHub gists containing generally used seed recordsdata. As an illustration for those who seek for “laravel us states seed file” you’ll discover fairly a couple of assets containing helpful seed recordsdata. However even this can be a tedious course of, as a result of you’ll want to kind by the outcomes, discover code that meets your wants, and probably modify it a bit additional to completely fit your utility. Absolutely there’s a higher means?
Land Your Dream Information Analyst Job in 2023!
Be taught to code with Treehouse Techdegree’s curated curriculum filled with real-world initiatives and alongside unbelievable pupil assist. Construct your portfolio. Get licensed. Land your dream job in tech. Join a free, 7-day trial at present!
Begin a Free Trial
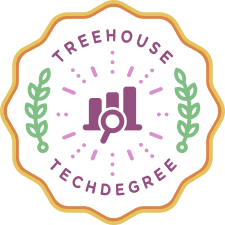
Enter ChatGPT
Utilizing ChatGPT you may generate these seed recordsdata utilizing a easy immediate. Let’s head over to https://chat.openai.com/ and create the identical seed file as I introduced a second in the past. I’ll use the next immediate:
Please generate a Laravel seed file containing the 2 digit
abbreviation and and identify of every US state. Simply present the
code with out clarification.
ChatGPT responded with:
<?php
use IlluminateDatabaseSeeder;
use IlluminateSupportFacadesDB;
class StatesTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public operate run()
{
$states = [
['abbreviation' => 'AL', 'name' => 'Alabama'],
['abbreviation' => 'AK', 'name' => 'Alaska'],
['abbreviation' => 'AZ', 'name' => 'Arizona'],
['abbreviation' => 'AR', 'name' => 'Arkansas'],
['abbreviation' => 'CA', 'name' => 'California'],
['abbreviation' => 'CO', 'name' => 'Colorado'],
['abbreviation' => 'CT', 'name' => 'Connecticut'],
['abbreviation' => 'DE', 'name' => 'Delaware'],
['abbreviation' => 'FL', 'name' => 'Florida'],
['abbreviation' => 'GA', 'name' => 'Georgia'],
['abbreviation' => 'HI', 'name' => 'Hawaii'],
['abbreviation' => 'ID', 'name' => 'Idaho'],
['abbreviation' => 'IL', 'name' => 'Illinois'],
['abbreviation' => 'IN', 'name' => 'Indiana'],
['abbreviation' => 'IA', 'name' => 'Iowa'],
['abbreviation' => 'KS', 'name' => 'Kansas'],
['abbreviation' => 'KY', 'name' => 'Kentucky'],
['abbreviation' => 'LA', 'name' => 'Louisiana'],
['abbreviation' => 'ME', 'name' => 'Maine'],
['abbreviation' => 'MD', 'name' => 'Maryland'],
['abbreviation' => 'MA', 'name' => 'Massachusetts'],
['abbreviation' => 'MI', 'name' => 'Michigan'],
['abbreviation' => 'MN', 'name' => 'Minnesota'],
['abbreviation' => 'MS', 'name' => 'Mississippi'],
['abbreviation' => 'MO', 'name' => 'Missouri'],
['abbreviation' => 'MT', 'name' => 'Montana'],
['abbreviation' => 'NE', 'name' => 'Nebraska'],
['abbreviation' => 'NV', 'name' => 'Nevada'],
['abbreviation' => 'NH', 'name' => 'New Hampshire'],
['abbreviation' => 'NJ', 'name' => 'New Jersey'],
['abbreviation' => 'NM', 'name' => 'New Mexico'],
['abbreviation' => 'NY', 'name' => 'New York'],
['abbreviation' => 'NC', 'name' => 'North Carolina'],
['abbreviation' => 'ND', 'name' => 'North Dakota'],
['abbreviation' => 'OH', 'name' => 'Ohio'],
['abbreviation' => 'OK', 'name' => 'Oklahoma'],
['abbreviation' => 'OR', 'name' => 'Oregon'],
['abbreviation' => 'PA', 'name' => 'Pennsylvania'],
['abbreviation' => 'RI', 'name' => 'Rhode Island'],
['abbreviation' => 'SC', 'name' => 'South Carolina'],
['abbreviation' => 'SD', 'name' => 'South Dakota'],
['abbreviation' => 'TN', 'name' => 'Tennessee'],
['abbreviation' => 'TX', 'name' => 'Texas'],
['abbreviation' => 'UT', 'name' => 'Ut
Note how the output abruptly stops prior to finishing the output. This is because we’ve hit ChatGPT’s currently imposed 3,000 character limit. This restriction will almost certainly change in the future so you might not run into this issue, however if so you can use this prompt to coerce ChatGPT into gracefully continuing:
continue writing the code in last response
ChatGPT will pick right up where it left off, finishing the snippet:
['abbreviation' => 'UT', 'name' => 'Utah'],
['abbreviation' => 'VT', 'name' => 'Vermont'],
['abbreviation' => 'VA', 'name' => 'Virginia'],
['abbreviation' => 'WA', 'name' => 'Washington'],
['abbreviation' => 'WV', 'name' => 'West Virginia'],
['abbreviation' => 'WI', 'name' => 'Wisconsin'],
['abbreviation' => 'WY', 'name' => 'Wyoming'],
];
DB::desk('states')->insert($states);
}
}
From right here you may copy each snippets into your seed file and proceed as desired.
Refining ChatGPT Code
Suppose you realized you made a mistake instructing ChatGPT to make use of abbreviation
and identify
attributes for the seed entries, and as an alternative meant to make use of abbr
for the abbreviation. Moderately than ask ChatGPT to regenerate the enter seed file you may say:
Please modify the $states array to make use of abbr as the important thing as an alternative of abbreviation. Return solely the states array and never your complete seed file.
ChatGPT returns:
$states = [
['abbr' => 'AL', 'name' => 'Alabama'],
['abbr' => 'AK', 'name' => 'Alaska'],
['abbr' => 'AZ', 'name' => 'Arizona'],
['abbr' => 'AR', 'name' => 'Arkansas'],
['abbr' => 'CA', 'name' => 'California'],
['abbr' => 'CO', 'name' => 'Colorado'],
['abbr' => 'CT', 'name' => 'Connecticut'],
['abbr' => 'DE', 'name' => 'Delaware'],
['abbr' => 'FL', 'name' => 'Florida'],
...
Producing Dynamic Seed Information with ChatGPT
It’s also possible to use ChatGPT to generate seed information dynamically. Most programming languages assist this means by a third-party open supply dependency. See the Instructor’s notes for a couple of examples. Within the case of PHP that third-party dependency known as Faker. ChatGPT occurs to grasp Faker and so we will instruct it to make use of Faker to generate 25 customers:
You're a Laravel programmer. Create a seed file that makes use of the Faker library to seed 25 customers right into a desk named customers. Every person ought to include a first_name, last_name, and electronic mail area.
ChatGPT will reply with:
<?php
use IlluminateDatabaseSeeder;
use IlluminateSupportFacadesDB;
use FakerFactory as Faker;
class UsersTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public operate run()
{
$faker = Faker::create();
for ($i = 0; $i < 25; $i++) {
DB::desk('customers')->insert([
'first_name' => $faker->firstName,
'last_name' => $faker->lastName,
'email' => $faker->unique()->safeEmail,
]);
}
}
}
If after producing the code you’re reminded that your customers desk doesn’t break the customers’ names into separate first and final identify fields, you may ask ChatGPT to revise the code:
Please revise your code so every person has a single area for the primary and
final identify as an alternative of separate fields first_name and last_name.
ChatGPT will reply with the next:
<?php
use IlluminateDatabaseSeeder;
use IlluminateSupportFacadesDB;
use FakerFactory as Faker;
class UsersTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public operate run()
{
$faker = Faker::create();
for ($i = 0; $i < 25; $i++) {
$fullName = $faker->identify;
$electronic mail = $faker->distinctive()->safeEmail;
DB::desk('customers')->insert([
'name' => $fullName,
'email' => $email,
]);
}
}
}
On this response I don’t like how ChatGPT first declared $fullName
and $electronic mail
variables, as a result of the code will likely be a tad cleaner if we simply instantiate the identify
and safeEmail
strategies straight contained in the insert
methodology. Let’s ask it to revise the code:
Please revise the code to name $faker object strategies inside
the insert methodology as an alternative of first instantiating variables for the identify and electronic mail.
Its response:
<?php
use IlluminateDatabaseSeeder;
use IlluminateSupportFacadesDB;
use FakerFactory as Faker;
class UsersTableSeeder extends Seeder
{
/**
* Run the database seeds.
*
* @return void
*/
public operate run()
{
$faker = Faker::create();
for ($i = 0; $i < 25; $i++) {
DB::desk('customers')->insert([
'name' => $faker->name,
'email' => $faker->unique()->safeEmail,
]);
}
}
}
Nice!
Conclusion
Instruments like ChatGPT and GitHub Copilot can actually supercharge your productiveness by serving to you to rapidly knock out tedious code and information units that in any other case would have required a good bit of Googling, copying, pasting, and reformatting. When you discovered this weblog submit helpful I invite you to join a free seven day Treehouse subscription to be taught extra about ChatGPT, AI, LLMs, information science, and extra!