Goal-C is an object-oriented programming language. There are two key parts of the programming language, together with the Goal-C class and the item. Let’s dive deeper into these parts so you possibly can higher perceive the fundamentals.
This text is the third a part of a sequence. You should definitely take a look at The Newbie’s Information to Goal-C: Language and Variables and The Newbie’s Information to Goal-C: Strategies for all the small print.
A Fast Refresh: What Is Goal-C?
Goal-C is an “object-oriented” programming language, however what does that imply? In an earlier submit, I described how “an object-oriented language is constructed across the idea of objects.” By that, I imply the programming language is utilized in such a method that real-world objects could be represented in code in an intuitive method that is smart.
As is usually the case, that is greatest illustrated with an instance. Let’s think about that we’re engaged on an app that permits customers to order pizzas from an area restaurant.
The person’s order is an instance of an “object.” It has properties, just like the person’s title, telephone quantity, and ordered objects. Every merchandise, like a pizza, is one other “object” with its personal properties, like measurement and toppings.
The properties are represented a sure method in code and could be accessed all through the app. This provides the code an organized construction that turns into extra obvious with use and observe.
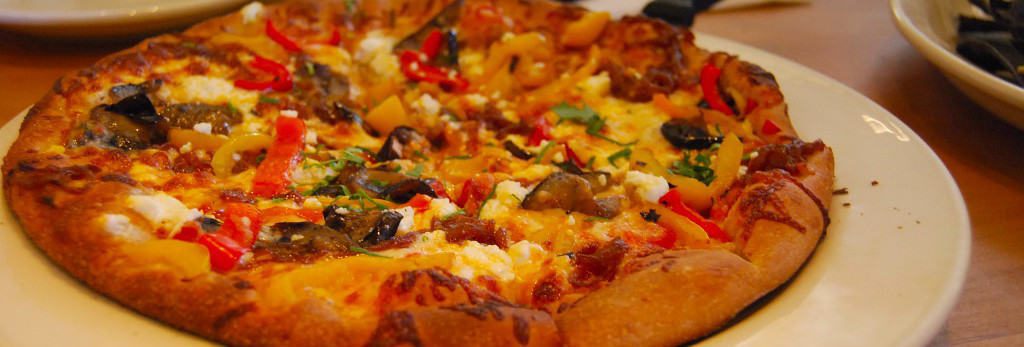
Something, bodily or summary, could be as an “object” in code. (ninacoco/Flickr)
These objects can even have behaviors related to them. With the order instance, it may be submitted or canceled. These two behaviors could be represented as “strategies” in code, which you may bear in mind from one other earlier submit.
In code, this could be represented as the next, which creates a easy order for Ben, provides a pepperoni pizza, and submits the order to the shop:
Order *order = [[Order alloc] init];
order.title = @"Ben";
[order.items addObject:[Pizza initWithToppings:@"Pepperoni"]];
[order submit];
Why Does Object-Oriented Programming Matter?
Programmers detest inefficiency and have spent many years establishing conventions and patterns for software program improvement that enhance effectivity in several methods.
Object-oriented rules permit for higher use, group, and understanding of code. It separates code into modules that may be simply reused or modified. Plus, it ensures the code is simpler to grasp for individuals who use it.
What Is an Goal-C Class and Object?
Typically, information of code in Goal-C are organized as courses. An Goal-C class is often represented by two information: a header file and a corresponding implementation file.
A category is supposed to outline an object and the way it works. On this method, an Goal-C class is sort of a blueprint of an object. Lessons outline issues about objects as properties, and talents of the item are outlined as strategies.
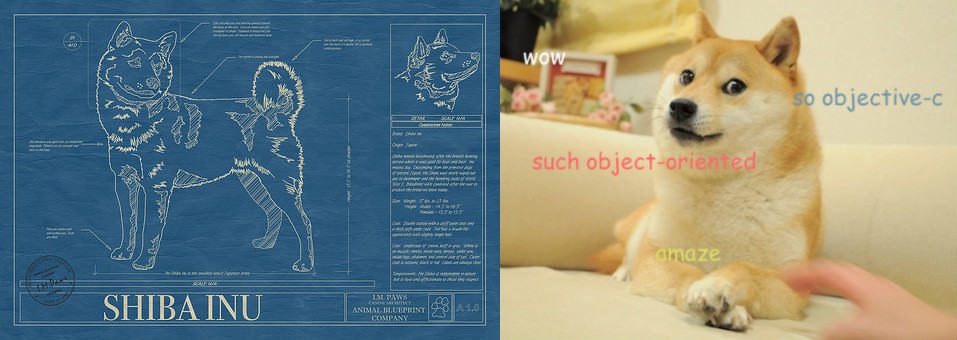
A category is sort of a blueprint for an object. (wondermonkey2k/Flickr)
Header Recordsdata in an Goal-C Class
The header file (with the file extension .h) defines the category and every little thing about it for the world to know. The thought is to separate the definition of a category from its nitty-gritty implementation particulars.
On this case, the header file acts because the interface to the remainder of your program. Your different code will entry every little thing a couple of class based mostly on what’s made obtainable in its interface, together with obtainable properties and strategies.
Let’s check out a category that could possibly be used to symbolize a easy connection to an internet site. This easy object has one property, a URL, and two strategies: “join” and “canHandleRequest.” The header file may look one thing like this:
UrlConnection.h
01 #import <Basis/Basis.h>
02
03 @interface UrlConnection : NSObject
04
05 @property NSString *url;
06
07 - (void)join;
08 + (BOOL)canHandleRequest:(NSString *)kind forUrl:(NSString *)url;
09
10 @finish
The primary line is what is called an import assertion. That is used to import required information that the code inside this class wants. On this instance, we’re importing the Basis courses, which embody NSObjects like NSString, NSArray, and so on. A category can have many import statements, every by itself line.
There’s one fast factor to level out about import statements: Typically you will note the names of issues in angle brackets, like this instance, and different instances you’ll see the names in double quotes, like #import "PizzaViewController.h"
.
Angle brackets inform the compiler to seek for the code to import in sure system paths, whereas the double quotes inform it to look inside the present undertaking.
The following line (line 3) is the interface declaration. It begins with @interface
and ends with @finish
on line 10. These particular markers designate the start and finish of the category interface definition. After @interface is the title, so the title of this instance class is “UrlConnection.” The title right here should match the title used within the .h and .m information.
Up subsequent on line 3 is a colon and one other title. The colon signifies that the following title is the father or mother class of this class. Object-oriented programming languages have an idea known as inheritance that’s used to share properties and strategies from one class to a different.
On this instance, our UrlConnection class is inheriting from the NSObject class, which is Goal-C’s generic object class. NSObject defines some very primary construction about objects in Goal-C, however courses can inherit from every other class.
For instance, we might create one other class named YouTubeUrlConnection that inherits from this UrlConnection class, and the interface declaration would appear to be this:
@interface YouTubeUrlConnection : UrlConnection
Properties
The following line within the class (line 5) declares a property of the category known as “url.”
@property NSString *url;
Properties, bear in mind, are information objects concerning the object. On this case, it will be the precise URL that this object will likely be making an attempt to connect with. Properties are declared with the particular @property
key phrase and finish with the semicolon.
After @property, we’ve got an everyday variable declaration that features its information kind (NSString on this case) and title (“url”).
I also needs to level out that properties can have attributes which are outlined inside parenthesis after the @property key phrase:
@property (nonatomic, sturdy) NSString *url; // Instance attributes
These are added to indicate different objects how you can work together with the property and to inform the compiler how you can mechanically create “getter” and “setter” strategies that can be utilized to set or retrieve the worth of the property.
Strategies
After the properties of a category, the strategies (assume habits) are often listed. Strategies are used to arrange our code into reusable (and comprehensible) chunks that save us lots of time and vitality.
On traces 7 and eight in our instance, we’ve got two strategies listed:
- (void)join;
+ (BOOL)canHandleRequest:(NSString *)kind forUrl:(NSString *)url;
Discover that these are simply the names of the tactic. This is called the technique signature. It additionally tells us what sort of information will likely be returned (if any), and what parameters could be required to name it.
The join
technique doesn’t have any parameters, nor does it return something. The canHandleRequest
technique returns a BOOL worth and has two parameters, each NSStrings.
Occasion vs. Class Strategies
Methodology declarations start with both a minus (-) or a plus signal (+). The minus signal signifies that this technique is an occasion technique.
Which means that an occasion of the category should be obtainable to name this technique. Or, in different phrases, it signifies that in our code we have to be utilizing an object constituted of this class, like if we allotted and initialized a UrlConnection object as a variable.
Class strategies (starting with the plus signal), can be utilized just by referencing the category. No occasion of the category is required. We are able to name a category technique anyplace in our code so long as the header file for the category is imported.
Let’s check out an instance of utilizing this UrlConnection class:
01 BOOL canHandleIt = [UrlConnection canHandleRequest:@"GET"
02 forUrl:@"http://www.teamtreehouse.com"];
03
04 if (canHandleIt) {
05 UrlConnection *connection = [[UrlConnection alloc] init];
06 connection.url = @"http://www.teamtreehouse.com";
07 [connection connect];
08 }
On traces 1 and a couple of we set a BOOL variable utilizing the canHandleRequest
technique. Sure, technique calls can span a couple of line if we wish! It’s as much as us.
Discover that no occasion of UrlConnection has been declared but, however we reference the tactic utilizing the category title.
On line 6 we’ve got a UrlConnection variable named “connection.” We use this variable, an occasion of the UrlConnection class, to name the join technique.
We aren’t allowed to jot down [connection canHandleRequest:@"GET"]
, nor can we write [UrlConnection connect]
.
Implementation Recordsdata within the Goal-C Class
The opposite half of a category is the implementation file (with the file extension .m). That is the place the magic occurs and the place the soiled work and the heavy lifting happen.
Implementation information implement all these issues that we declare to be obtainable within the header information. Each technique that we are saying an Goal-C class has should be outlined with all its vital code within the implementation file.
Persevering with with our UrlConnection instance, the next is a shortened model of what the implementation file may appear to be:
UrlConnection.m
01 #import "UrlConnection.h"
02
03 @implementation UrlConnection
04
05 - (void)join {
06 /* In right here can be code to try a connection to the
07 * specified URL, whereas presumably dealing with connection errors.
08 */
09 }
10
11 + (BOOL)canHandleRequest:(NSString *)kind
12 forUrl:(NSString *)url {
13 /* And in right here can be code to see if the given URL handed
14 * in is able to dealing with the HTTP request kind specified
15 * by the "kind" parameter. It's going to return YES or NO.
16 */
17 }
18
19 @finish
Discover that the header file should be imported (line 1). As a result of these are two separate information, the compiler should be advised the place to seek out the header file that belongs to this implementation file.
As we noticed earlier, the double quotes are used on this line to point that the file ought to be situated in the identical undertaking as this implementation file.
Subsequent, on line 3, we start the precise implementation of the category with the @implementation
key phrase. This correlates to the @interface
key phrase for the header file. And in the identical method, the implementation ends with the @finish
key phrase on line 19.
Primarily, we see technique definitions contained in the implementation. In our instance, we’ve got two strategies, that are outlined right here. Discover that the signature for the strategies is the very same as what we noticed within the header file.
What’s totally different is that the physique of the tactic, the code that makes it up, can be proven right here as a bunch of statements inside curly brackets that instantly observe the tactic signature.
Now, we would not have any code on this instance as a result of that isn’t what we’re specializing in right here. However the feedback supplied give an thought as to what the Goal-C statements contained in the strategies will likely be doing.
Study Extra About Programming With Treehouse
The aim of this information was that can assist you higher perceive the Goal-C class and object so you should use them as you code. Realizing how you can arrange and construction your objects is an artwork that even skilled programmers are consistently refining.
If you wish to dive deeper into programming, take a look at Treehouse’s numerous Techdegrees, tracks, and programs. Get began right this moment by signing up for a free trial of Treehouse. Completely satisfied coding!
*Shiba Inu blueprint courtesy of wondermonkey2k and pizza picture courtesy of ninacoco beneath the Inventive Commons license.