If there solely was a approach to create spectacular JavaScript animations simply and with out having to fret about browser compatibility… oh wait, there’s one! Or maybe multiple. At present, you’ll see how you can develop such animations with the GreenSock Animation Platform, or GSAP. Why did I select this one? How does it actually work? What can it do for you? Let’s discover out.
Have you ever ever labored on a venture which required you to create advanced animations in a JavaScript utility that depends on frequent consumer interactions?
I simply have. At present I’m going to let you know extra a couple of software that made this expertise way more fascinating.
The problem
My newest expertise with JavaScript animations happened when one in all our shoppers requested my crew to develop a brand new function for his or her extremely safe utility. It was purported to be one thing akin to a lock display.
If you happen to ever used a banking utility in your life, you undoubtedly acknowledge the display that exhibits up if you end up logged out of your session as a consequence of a interval of inactivity.
This one labored very like that, aside from one small element.
The distinction was that as an alternative of being logged out completely, you get a lock display with an choice to return to the app by writing your PIN quantity.
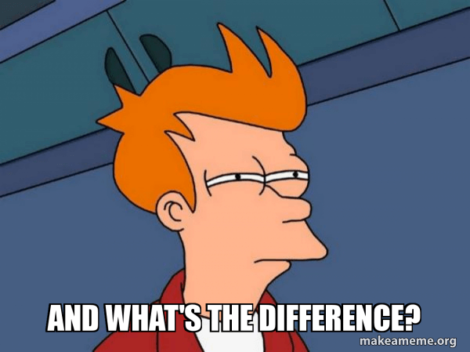
I admit that in apply, it quantities to just about the identical factor for the consumer. From a technical standpoint, it’s a totally different conduct as you don’t must sort in your full credentials.
Apart from safety, the shopper significantly valued one other facet of their app – its look. The venture was designed with nice care and a spotlight to element. It included advanced and effectively–optimized animations. The lock display was to be no totally different.
What does it imply?
It signifies that I wanted to give you some actually nice-looking JavaScript animations!
Why the GSAP library?
JavaScript-based animations are usually not a simple topic. The very first thing that got here to my thoughts was to discover a library that would make the event sooner. Caring for numerous browser inconsistencies in rendering JS animations was one other essential goal. Lengthy story quick, I picked the GreenSock Animation Platform (GSAP). ?
GSAP is a strong JavaScript library and a strong JavaScript toolset that makes it simpler to create skilled grade animation. You should utilize it to design all types of JS animations, starting from easy manipulations of particular person DOM components to advanced results that contain a number of segments triggered at particular moments. Such results embody:
- Progressively revealing or hiding SVG strokes to attain the impact of drawing in real-time (the DrawSVGPlugin plugin).
- Turning (morphing) one SVG form into one other easily (MorphSVGPlugin).
- Shifting generic objects alongside a predetermined path (MotionPathPlugin).
GSAP provides you exact management over CSS properties, making it attainable to create skilled grade animations. It additionally supplies superior playback controls and accessibility pleasant animations. This explicit library met the necessities of the venture better of all out there choices for a few causes:
- It makes it attainable to implement all forms of animations that had been related to the venture.
- It may animate something JavaScript, that’s, any numerical property of JavaScript or a given object.
- GSAP is so complete that it may well animate any CSS/JS property supported by a given browser.
- After which, there’s the group. The authors created a dialogue discussion board that over time produced a bigger consumer base. It’s actually vibrant, full of individuals prepared and capable of assist.
- And contemplating the recognition of GSAP, there aren’t that many points you might come throughout that weren’t already addressed earlier than.
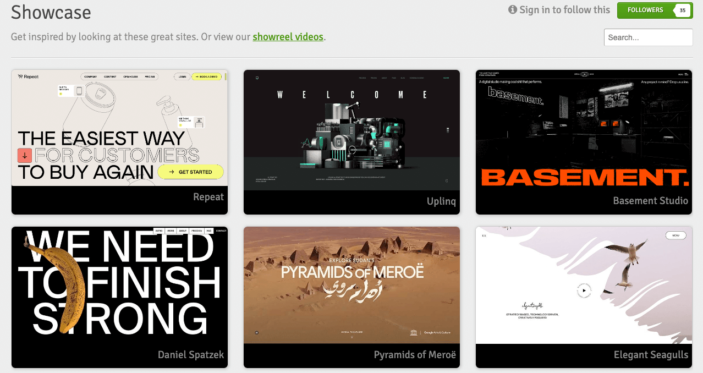
You possibly can add GSAP to your venture in numerous methods – by importing native information, by way of npm, or by utilizing CDN (“cdnjs.cloudflare.com ajax libs gsap”).
GSAP animations weren’t the one various I thought of.
GSAP alternate options
One other answer I thought of is anime.js. It might need nice potential, however its downside is the shortage of steady help for the library. The software program has not been up to date for nearly 2 years. And whereas it nonetheless works simply tremendous, and there’s no clear indication that the library was deserted, I can’t assist however be cautious of that scenario.
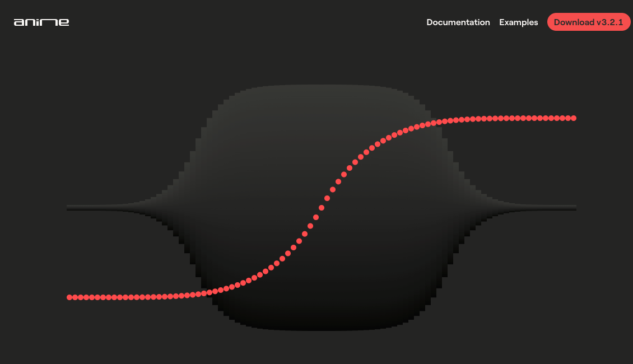
When engaged on giant and scalable methods, such because the one I’m tackling on this case research, you should have full confidence in all of the third-party options you utilize.
In that regard, GSAP was a transparent winner when in comparison with alternate options reminiscent of anime.js or Animate css.
Helpful GSAP assets
As I mentioned, GSAP supplies a number of assets that make your introduction to the library smoother. I extremely suggest you strive these:
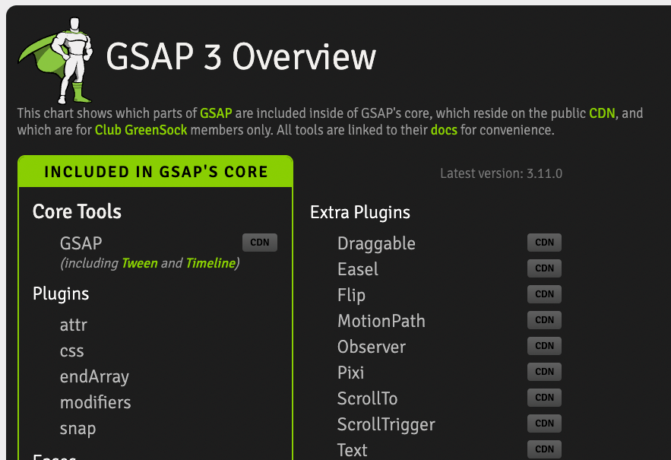
Alright! With that mentioned, I assume we will lastly check out the library itself.
How does GSAP work? GSAP tutorial
Earlier than I get again to the venture, I’m going to present you a fast overview of the idea so as to create animations like this your self.
Concept
The tween
Once you begin with GSAP, one of many first ideas you study is the tween. That is the constructing block of any GSAP-based work.
The tween is one unit of animation. You possibly can provoke it in 3 ways:
- gsap.to() – all you establish are the ending CSS values your object is to be animated into.
- gsap.fromTo() – you establish each beginning and ending CSS values of your animations.
- gsap.from() – you solely decide beginning values (the ending values can be inherited from default CSS types).
I sorted the checklist above, ranging from those who I really feel are used probably the most for tweens.
Let’s check out an instance of a tween based mostly on the gsap.to() perform.
A HTML ingredient is the primary argument of the perform. To do the identical in React, you’ll usually flip to useRef. Along with the ingredient itself, you may also reference an ID or HTML class. That offers you loads of choices to get to components wherein including a ref is troublesome. A great instance of such components may very well be SVGs imported from property and used as common React elements.
The second argument used here’s a number of animation properties. The entire checklist of properties clearly contains the entire CSS properties. As well as, GSAP provides a number of customized properties. It additionally contains callbacks, which function one thing of a bridge between the world of animation and JavaScript. Due to callbacks, animation occasions can set off some secondary results.
Probably the most fascinating and most used properties is autoAlpha. When set to 0, it combines the results of opacity set to 0 and visibility set to hidden.
Timeline animations
Let’s say you need to develop a extra advanced animation with GSAP. You need to begin by studying extra about timeline animation, which is basically a cluster of tweens. GSAP makes it attainable to make use of superior sequencing in a timeline to attain nice management over particular person tweens. Let’s check out an instance of such superior sequencing.
Let’s modify the earlier instance by breaking it into two separate phases. Every stage is a separate tween and the entire piece of code is a timeline.
Not too arduous, isn’t it?
You might need observed an extra argument within the final tween – +=0.5. Once you use tweens inside a timeline, you may add time modifiers. The one above signifies that the animation will execute half a second after the earlier one is accomplished.
Again to the venture – a sensible GSAP case research
That’s it for the essential concept. At this level, you need to be greater than acquainted sufficient with GSAP to dive deeper into my venture. To make it slightly simpler, nonetheless, let’s put apart the problem of safety and focus completely on the animations.
However what sort of animation tutorial would it not be with out one thing so that you can click on at? To make it extra fascinating, I ready a dwell animation instance in CodeSandbox. I extremely encourage you to play with it a bit.
In an actual venture, I take advantage of the GSAP bundle. This time, I’ll use the gsap-trial bundle that’s solely supposed for experimentation. It contains numerous bonus information, a few of which you usually must pay for. I’ll get again to the topic of plugins later. For now, let’s concentrate on the code.
On this simplified instance, the display is blocked if you click on the button.
The mechanism is as follows:
- Once you click on the button, you set off the animation.
- The curtains present up.
- Then, the padlock locks.
- The keyhole turns crimson.
- With a view to unlock it, you click on the padlock once more.
- The animation is triggered once more, however it’s now in reverse.
All the course of takes place contained in the ScreenLocker element.
For starters, you should declare your refs after which insert them into correct tags.
Inside my ref, I put not solely my HTML and SVG components, however the timeline as effectively. Due to that, it gained’t need to be created a number of instances when the element’s logic will get extra advanced. The timeline is ready to paused by default in order that it doesn’t launch proper after it’s outlined.
Subsequent, I must set the preliminary values of all components. It’s essential to do it at first to have just one supply of reality concerning these values. I actually can’t stress sufficient simply how essential it’s. Oftentimes, one in all my teammates would set a CSS worth, would NOT inform anybody about this, after which overlook about the entire thing the subsequent day. After which – the animation misbehaves and no person is aware of why. A single supply of reality (in time and within the flesh) actually does make a distinction.
I must make it possible for my refs are usually not null throughout the initialization.
Then, I can set all of the required properties. As you may see within the final gsap.set, the values are usually not restricted to strings and numbers. A perform is one other widespread choice. You should utilize it to execute some calculations based mostly on different sources.
As you may see, an array of components can be allowed. It provides you much more choices. For instance, you may set totally different values relying on whether or not the array ingredient is odd and even.
On this instance, the curtain is to maneuver to the left for the primary ingredient (index=0) after which to the proper for the second ingredient (index=1).
Now, I’m going to implement the lock display timeline.
It’s important for the useEffect or useLayoutEffect hooks, wherein I outlined the timeline, to execute solely as soon as. In any other case, every subsequent callback execution will trigger the timelines to stack up.
The timeline consists of 5 tweens:
- The curtains transfer inwards.
- Concurrently (because of the < modifier), the padlock exhibits up.
- 0,2 second after the earlier tween concludes, the padlock deal with goes again to the closed place after which bounces again barely, simulating how a padlock usually closes. This rebounding impact is created with the again.in(3) easing.
- Lastly, the keyhole turns crimson to point that the display is now locked.
The useEffect hook incorporates extra data on the conduct of the timeline:
In case of issues with React 18 and timelines stacking up within the dev mode, it’s advisable to show off the StrictMode.
And that’s it for the venture! As soon as you recognize all of the fundamentals, you may be effectively outfitted to make animations reminiscent of these I confirmed you. And everytime you run into hassle, you need to check out the docs. It’s very complete and even features a widespread errors part. If you happen to ever spent any significant time making an attempt to make a JavaScript animation work, you’ll actually respect it.
Prolong GSAP capabilities with plugins
This tutorial wouldn’t be full with no phrase or two about plugins. In any case, whereas I intentionally didn’t use them in my easy instance, they’re an especially essential and helpful facet of utilizing GSAP in apply.
There are two plugins that I discover significantly fascinating:
ScrollTrigger
This appropriately named GSAP plugin makes it attainable to set off sure animations and callbacks if you execute corresponding scroll occasions. For instance, if you scroll your manner into a selected space of your app, depart it, or enter it from the highest.
Draggable
One other sensible plugin is Draggable. And it’s one other one which bought a extremely applicable identify, because it focuses on producing the dragging impact for HTML components. All it takes is a brief little bit of code:
With this straightforward hook, you can also make every HTML ingredient draggable. Right here is how you need to use it in apply:
The hook returns a ref, which you’ll be able to insert into the ingredient of your HTML file.
That’s the gist of it. After all, you may develop this idea by altering how the draggable impact is meant to work. You should utilize it:
- On the X-axis.
- On the Y-axis.
- Or by rotating a single ingredient.
Draggable works effectively with the paid Inertia plugin. When mixed collectively, you may enrich the dragging impact with spectacular finishes, reminiscent of having a component glide to a cease in a easy manner. You possibly can take a look at it with gsap-trial.
Classes realized – why and when to make use of the GSAP library
GSAP has a number of advantages:
- It’s actually intuitive and simple to make use of.
- The animations made with GSAP are well-optimized out-of-the-box.
- The group is large and actually useful.
- The docs is an actual standout.
Nonetheless, that doesn’t imply that GSAP is your best option for each venture.
Use it for apps that require a number of components, excessive efficiency animations, the necessity for movement paths, and anything that permits entrance finish builders to attain superior results simply.
I might not suggest it for apps that solely sport probably the most fundamental animations. In such tasks, the overhead brought on by together with GSAP is not going to be definitely worth the contribution it makes. In any case, a library as strong and highly effective as this one has to weigh a bit. Within the case of GSAP, the load is about 60kB when minified and 25kB when minified and gzipped. And that’s for the essential model with out plugins. It’s considerably corresponding to the JavaScript utility library Lodash.
Along with that, for easy animations, the quantity of code generated with GSAP is likely to be larger than with pure CSS.
If all you need is so as to add a few fundamental results, you really want to research if including such a dependency is a good suggestion.
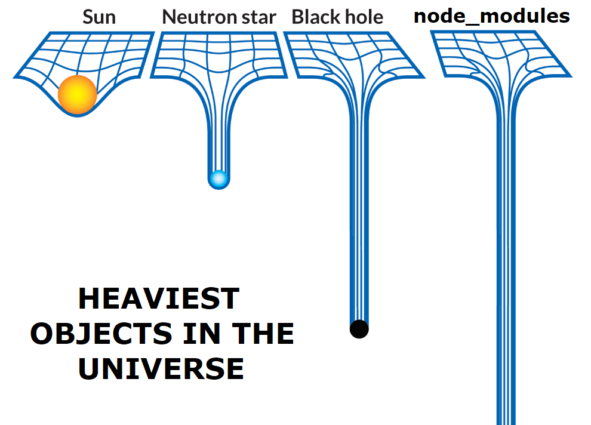
Apart from that, GSAP actually does ship on its guarantees, offering you with spectacular and well-optimized animations in your customized software program venture. It’s additionally actually enjoyable to make use of.
However don’t take me at my phrase – strive the GSAP sandbox and discover out for your self!