Consisting of plenty of applied sciences, frameworks, and providers, whereas serving a number of APIs and databases, the frontend layer’s complexity typically holds a candle to even essentially the most sturdy of backends and is de facto difficult to deal with. The dangerous information is that it’s unavoidable. The excellent news is you can come up with that mess utilizing correct design patterns. Learn how we developed an environment friendly and scalable system utilizing the Backend For Frontend sample with microservices.
We have now just lately labored on a challenge that supplied an ideal alternative to make use of the favored Backend For Frontend sample. However regardless of how fancy the know-how, it’s nothing with out enterprise context. Let’s begin with some primary details about the shopper.
Background
Let’s begin with some enterprise context.
The shopper
Again in 2018, we launched into a brand new challenge with Takamol Holding, a Saudi government-owned firm in command of creating an modern IT system meant to play a pivotal position within the digitalization of enterprise in Saudi Arabia, the labor market specifically. The aim was to serve quite a lot of completely different customers, each employers, and staff. As such, it required plenty of separate functionalities.
If you wish to be taught much more concerning the platform and enterprise context, we extremely suggest you learn our earlier article on the Qiwa digital transformation software program.
Ranging from now, we’re going to go extra technical and give attention to how we made it occur as builders.
What’s all of it about?
Because the platform was to serve a number of functions, a choice was made on the shopper’s finish to divide it right into a group of smaller sub-platforms. They’d be represented as tiles on the homepage of the service.
Every sub-platform represents a single enterprise operation, together with (however not restricted to):
- beginning a enterprise,
- signing a contract,
- transferring staff from one firm to a different.
The target right here was to maneuver all the providers to the web. The shopper had its personal API service, which communicated with the federal government’s database to finish numerous enterprise operations.
As The Software program Home group, our aim was to develop the visible layer of plenty of such particular person sub-platforms in addition to combine them with numerous providers of the shopper’s API.
Problem & answer
The complicated nature of the platform posed plenty of challenges.
The problem – be prepared for in the present day & tomorrow
From the beginning, we wished to make it possible for the platform could be well-equipped to take care of current and potential future points. We would have liked to plan it actually completely:
- Not solely does the system consists of varied sub-platforms, however the variety of platforms is anticipated to develop sooner or later. Therefore, scalability is of utmost significance.
- The variety of customers will develop too. The truth is, the plan is to serve all employers and staff in Saudi Arabia finally.
- Every sub-platform offers a separate service. A selected service could also be related only for among the complete platform’s customers (e.g. only for employers or simply for workplace employees.). Contemplating this, it’s simple to see why this division makes numerous sense from the enterprise perspective.
- Every sub-platform will talk with solely chosen providers of the shopper’s API – solely those it must do its job.
- The shopper cooperates with different exterior contractors. The structure makes it potential for them to give attention to one sub-platform assigned to them. The truth is, they don’t even have to concentrate on different sub-platforms’ existence more often than not.
Our reply – Backend For Frontend
We agreed the easiest way for the frontend to work with such an structure is to make use of the Backend For Frontend design sample, a preferred architectural sample, a substitute for the API Gateway sample. Its defining characteristic is that every separate frontend app has its personal devoted API, which communicates with exterior APIs. That manner, completely different frontend apps of various objective and construction, together with these for cell functions / cell interfaces or desktop net UI, can have their very own devoted backend providers.
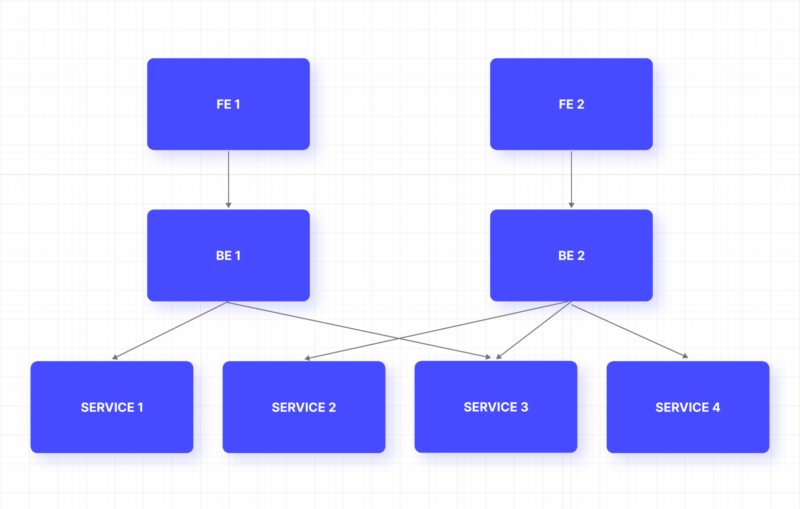
As you possibly can see within the diagram above, every “backend for frontend” communicates with numerous API providers underneath the hood. Every backend for frontend has three major capabilities:
- receiving responses in numerous codecs.
- managing and resolving errors it encounters.
- delivering the response to the frontend layer within the desired type (sometimes within the JSON format).
The backend app within the BFF sample is a defend for the frontend app. It protects it from errors and non-optimal response codecs from numerous exterior APIs.
Backend For Frontend in motion
Earlier than we get to the implementation, let’s take a more in-depth take a look at the BFF sample. It’s best utilized in very particular circumstances:
- tasks based mostly on the microservices structure,
- tasks that talk with exterior providers that require safety authorizations,
- tasks during which it’s fascinating for numerous separate groups to work independently on completely different elements of the system,
- and tasks, during which shopper apps name for separate APIs (e.g. separate APIs for cell apps, net apps, desktop apps, and so forth.).
It appears like our challenge matches the invoice nearly completely. Nothing’s stopping us from implementing Backend For Frontend!
Backend For Frontend implementation
We’re going to divide this part into the precise implementation and our ideas on the professionals and cons of BFF in tasks of this type.
BFF in our challenge
This diagram beneath depicts the implementation of the BFF sample in our challenge for 2 separate sub-platforms/providers (worker contract administration service and worker switch service).
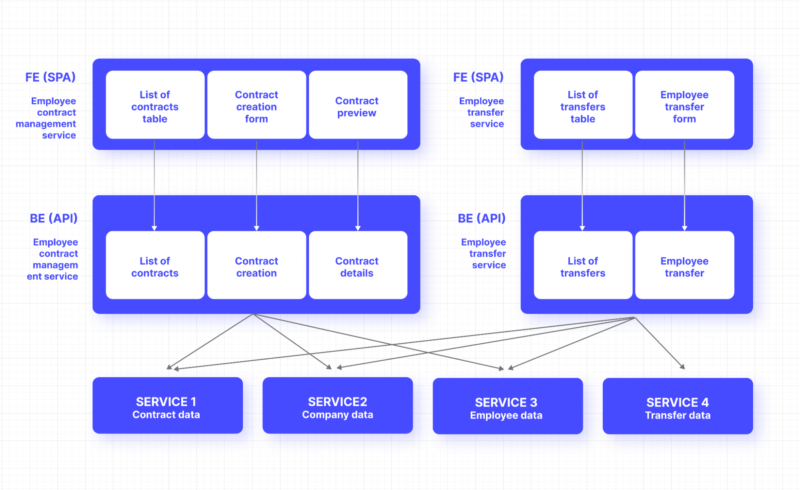
There are a number of BFFs or sub-platforms. The worker contract administration service is among the largest sub-platforms. It consists primarily of three views:
- a listing of contracts desk,
- a contact creation type,
- and a contract preview.
Every of those views communicates with a number of endpoints of a devoted API (optimized backend for frontend). The latter alternatively communicates with a number of exterior providers with the intention to move responses in a given format.
The opposite sub-platform additionally has a devoted API. It communicates with the identical exterior providers in addition to with an extra one – an worker switch knowledge service.
There are two primary conclusions to attract from it:
- The BFF API communicates solely with the providers it must work.
- The frontend apps don’t talk immediately with exterior providers. The latter is hidden from them and guarded with correct safety keys.
The elemental a part of the BFF sample right here is the contract made between the frontend app and its devoted backend app. It features a particular construction and format of frontend requests in addition to backend responses. The contract is impartial of the exterior providers. When the latter change, all that’s required is to switch the communication between the devoted backend (for frontend) and the exterior providers.
What did BFF give us? Backend For Frontend advantages
The Backend For Frontend sample offers plenty of advantages from the frontend perspective.
- No have to undergo the trouble of integrating with numerous exterior providers. The devoted backend handles all of the work.
- No want to switch something on the frontend aspect when exterior providers change.
- All of which means you can focus extra on sharpening your consumer interface.
There are additionally clear BFF advantages for the backend:
- All the knowledge dealing with logic is in a single place.
- Because the devoted frontend app is the one receiver of knowledge, you already know precisely what sort of knowledge to organize.
- Safety keys for exterior providers are saved fully within the devoted backend. They don’t seem to be obtainable in any manner in any respect to the frontend app.
As well as, going for the Backend For Frontend design offers numerous common advantages that welcome in nearly any challenge:
- The power to begin growth rapidly. In spite of everything, every group solely must know the codebase of its personal separate service. Very helpful when a brand new group takes over a challenge.
- The exact same modularity implies that you need to use completely different applied sciences for every separate service. You may choose one of the best know-how for the job, or just the one every group likes greatest.
- Every service is a greenfield challenge. There isn’t a legacy code. You might be free to make use of the newest model of frameworks or libraries for every service.
- Simplified refactoring – when there’s an error, you already know the place to search for it. And as soon as you discover it and take away it, you already know that it’ll not have an effect on different providers.
- Every service has a separate repo – it makes for an orderly and easy-to-browse-through challenge.
- Unbiased deployment of every frontend-backend pair, which reduces the variety of issues you’ll want to fear about when transport.
One of the best ways so that you can expertise the advantages of Backend For Frontend is by having a look on the code.
BFF sample advantages – a sensible instance
Let’s see how you need to use a devoted backend to make it possible for your app integrates simply with exterior providers.
The backend’s API sends a request payload to an exterior service. The payload consists of the ID and SECRET fields within the header. For safety causes, this knowledge can solely be saved within the backend. When the request is processed efficiently, the API receives the next HTTP 200 response:
When there is just one CompanyActivity, the exterior API may ship a single object as an alternative of an object desk. Nonetheless, due to the devoted backend, the response the frontend finally receives at all times has the identical formatting:
Within the browser, the response appears like this:
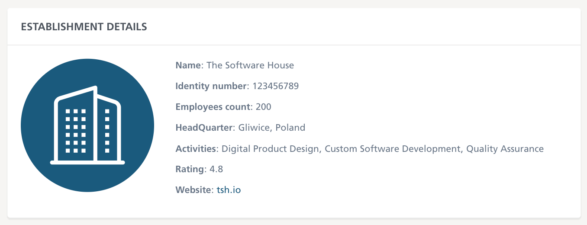
BFF shortcomings to account for
What about BFF cons? In the case of frontend:
- The event is prolonged as the method of integrating the backend app with exterior providers might be time-consuming.
So far as the backend goes, the most important downside with BFF is:
- Excessive danger of repeating items of code, particularly when the devoted backend apps typically group with the very same exterior providers.
Normal BFF cons come down specifically to:
- Modifying an present working characteristic could entail the necessity of adjusting the contract between the frontend app and its devoted backend.
- Since its devoted backend is impartial and may use completely different applied sciences, there’s a lack of cohesion and consistency by way of know-how decisions. It might pose upkeep points.
All of the cons of Backend For Frontend must do with the dimensions of the challenge. The bigger it’s, the much less of an issue they’re. Whenever you work on a big challenge like this one, you possibly can (and will) afford the additional setup work with the intention to reap the scalability advantages sooner or later. Whenever you do, the BFF sample will hardly have any cons for you.
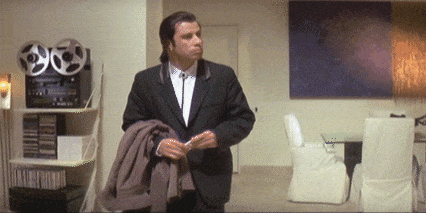
Within the length of the challenge, The Software program Home group recognized the shopper’s enterprise wants and the easiest way to narrate them to particular applied sciences and patterns.
Under, you possibly can see the challenge in numbers.
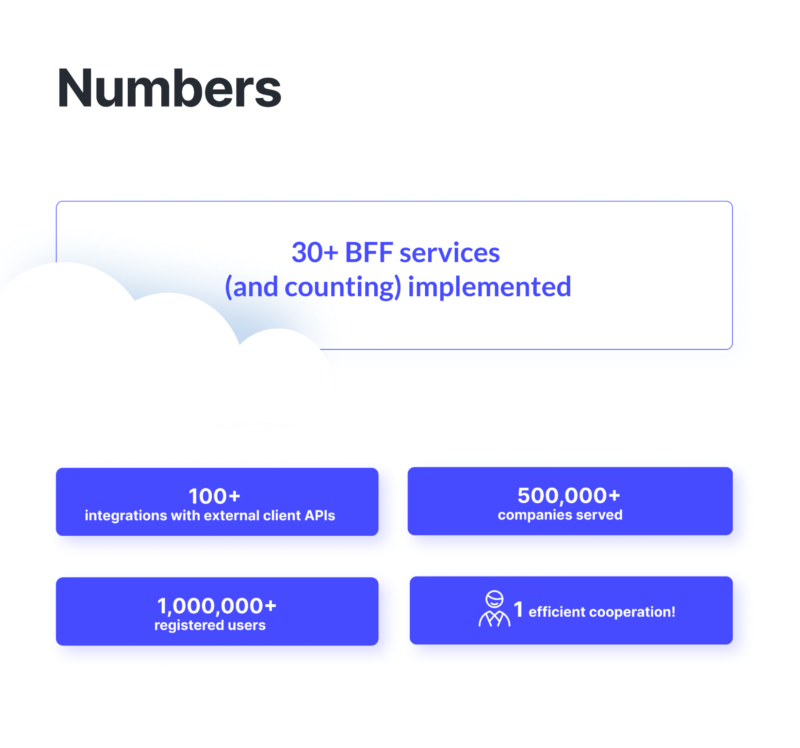
Our group labored principally in Node and React, having switched from Vue.js. The backend used Laravel. You may be taught extra a few wider technological context of the challenge within the beforehand talked about common Qiwa case examine.
Let’s now put our impact into tangible enterprise advantages.
Enterprise Implications
The challenge introduced enhancements to the shopper’s backside line of each quantitive and qualitative nature.
Quantitative impression
- By introducing so many providers, we made it potential for separate groups to proceed their work on them independently from each other.
- The challenge contributed to the digital transformation of the general public sector in Saudi Arabia – one of many said missions of our shopper.
Qualitative impression
The challenge will convey plenty of different advantages alongside the best way.
- It’s absolutely scalable, making it simple so as to add new options/providers sooner or later.
- It’s very steady. Since all of the providers are impartial of each other, even when one malfunctions, it won’t have an effect on different providers.
- It solves actual issues of Saudi employers and staff, making it potential for them to resolve many on a regular basis issues manner quicker.
Backend For Frontend is unquestionably fascinating, however the story isn’t actually concerning the BFF sample itself.
Conclusions – is BFF best for you?
The Qiwa problem has confirmed very profitable for each the shopper and us. We at all times find it irresistible once we get a brand new main problem from an organization now we have already been working with for a very long time – it’s proof of simply how wholesome and fruitful our cooperation is. And that’s how this backend service problem began.
Within the length of that challenge we managed to:
- diagnose the technological wants of the shopper based mostly on its enterprise necessities,
- discover the correct patterns and applied sciences to show the speculation into an precise working software with a number of providers,
- ship a high-performing scalable backend providers prepared for painless future expansions.
Whereas Backend For Frontend isn’t a cure-all, likelihood is that it could be simply what you’re searching for in your microservice structure.
A technique to ensure if that’s the case is…
… by consulting your challenge with the group at The Software program Home.