Net frameworks make constructing Node.js internet functions simpler. They take the guesswork out of dealing with requests and HTTP strategies. They supply dynamic HTML rendering, templates, and architectural patterns that make growing apps simpler. Over time, Categorical turned the Node.js internet framework of selection. However is it all the time the very best one? The creators of Fastify consider that it’s not the case. I used to be in a position to expertise Fastify first-hand throughout a current mission. Do I aspect with them or not?
Only a whereas in the past, my Node.js group and I labored on a mission of a reward system for gamers of assorted free-to-play on-line video games. The shopper needed to revamp the entire app dramatically. For all intents and functions, we had been to develop a complete new model of the system.
One of many major mission necessities was growing the pace of the system. The app had quite a lot of customers who carried out quite a lot of actions. The requests per second metric was of explicit significance.
Our pure framework of selection for Node.js-based was Categorical. It’s simple to study, very properly documented, and extremely versatile. However since pace was speculated to be such an enormous issue, we determined to take a better take a look at different choices as a way to make a correct pace comparability.
Quickly sufficient, we began contemplating a framework that bears a reputation that’s meant to make you immediately consider pace – Fastify.
What’s Fastify?
Fastify is a Node.js library that was created by Matteo Collina and David Mark Clements in late 2016. The newest model out there may be 4.7.0. If you’re within the launch historical past, examine the details about long-term help and corresponding launch notes.
In accordance with the creators:
Fastify was born out of a want to create a general-purpose internet framework that would supply a fantastic developer expertise with out compromising on throughput and efficiency.
Fastify is downloaded greater than 700,000 instances every week. At this second, it’s utilized in about 25,000 tasks. A number of corporations are already on board, together with giants corresponding to Microsoft. Listed here are among the companies that use Fastify.
Fastify efficiency – pace take a look at
When the shopper expressed their curiosity in prioritizing utility pace, we determined to check out Fastify. They are saying it is likely one of the quickest internet frameworks. Naturally, we couldn’t decide it up on fame alone.
We tried to search out as many analysis sources on the subject of Fastify as potential. Then, we determined to run exams on our personal.
To that finish, we used the wg/wrk benchmarking instrument. We in contrast Fastify and Categorical in 10 impartial exams. We anticipated Fastify to be sooner, however we would have liked detailed info to determine whether or not altering the Node.js framework is well worth the hassle.
The comparability included quite a lot of elements:
- requests per second,
- variety of requests served in 30 seconds,
- switch per second,
- common latency.
Right here is our Fastify take a look at information:
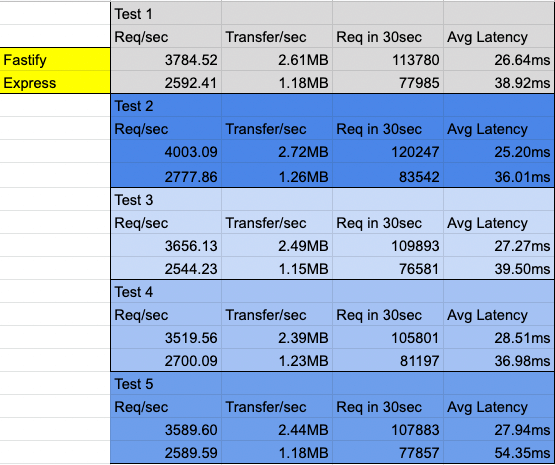
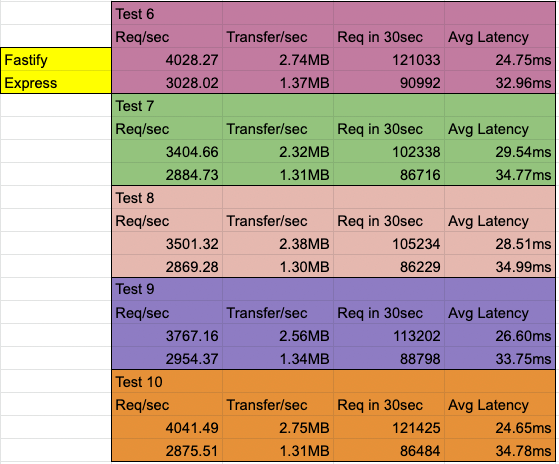
In each single take a look at, Fastify proved superior. On common, the framework carried out about 25 % higher than Categorical.
Our findings each stunned and impressed us. They influenced our choice to change to Fastify. Nonetheless, they had been hardly the one issue.
With the intention to clarify it totally, let’s take a better take a look at the framework.
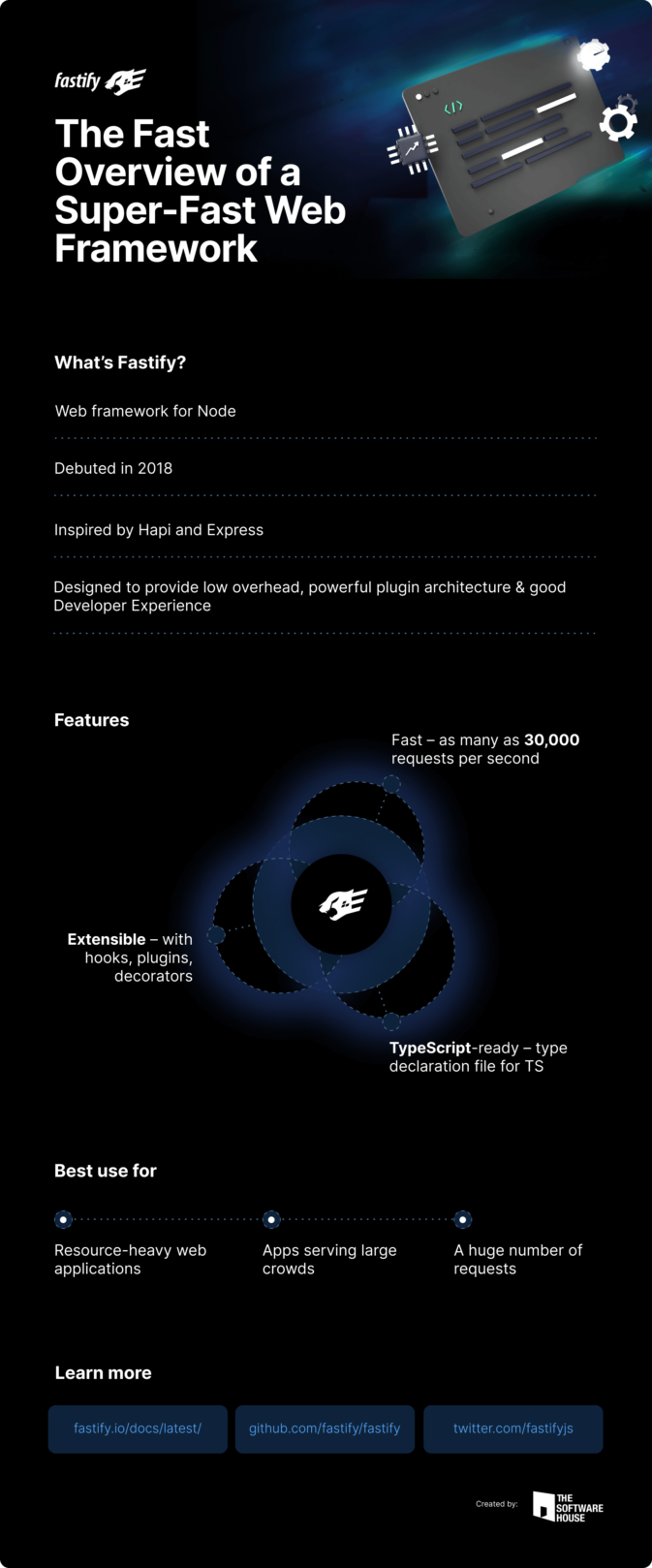
Why use Fastify?
Based mostly on our observations, Fastify’s benefits will be grouped into 4 classes.
Efficiency
We already talked about pace. However Fastify can also be extremely scalable, making the framework appropriate for each small and enormous tasks. The JSON format routinely analyzes Fastifiy’s features, guaranteeing quick routing of information packets throughout the community. That’s what makes the framework lightning-fast.
Maintainability
Fastify is a low overhead internet framework, which minimizes upkeep prices for your complete utility. Along with that, it’s an online framework extremely targeted on safety that ensures a system of automated safety and information validation.
Flexibility
Fastify helps TypeScript, cooperates with AWS Lambda, and communicates with its API by way of a GraphQL adapter. Consequently, it’s nice for internet utility improvement in addition to API interface improvement.
Ease of improvement
One in every of Fastify’s largest professionals in serverless functions is the convenience of improvement. In your native atmosphere, you’ll all the time run the Fastify utility straight, with out the necessity for any extra instruments, whereas the identical code can be executed in your serverless platform of selection with an extra snippet of code.
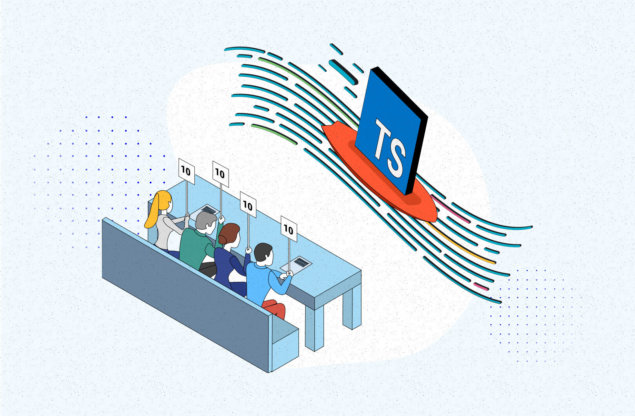
Do you assume that Fastify may very well be the know-how on your subsequent mission?
Why don’t you contact us to seek the advice of it?
Do you wish to effectively deal with your server’s assets whereas serving the very best variety of requests potential? Do you wish to do all that with out sacrificing safety validations and useful improvement?
Fastify was made for this state of affairs.
The mission I discussed earlier than is an ideal instance of such a state of affairs. The shopper anticipated the app for use by an infinite variety of folks. And since we needed the brand new model to be a particular improve of model one in relation to efficiency, altering Categorical to Fastify was a logical step.
When not to make use of Fastify?
Naturally, every thing has its professionals and cons – Fastify isn’t any exception. As of 2022, the framework shouldn’t be broadly used within the business. It’s being more and more adopted, however its use remains to be modest when in comparison with Categorical or Nest.
Along with that, being a reasonably younger mission, the documentation shouldn’t be as expansive as you would possibly anticipate from extra mature frameworks. The group help is so-so. For those who plan on utilizing a library corresponding to passport.js, you will discover that it doesn’t work with Fastify. Ensure that Fastify is appropriate with the entire different items of software program you wish to use.
If you’re an inexperienced developer in search of a job, you would possibly discover that only a few employers are presently in search of devs that know Fastify particularly. Consequently, you is likely to be higher served going for one thing extra mainstream.
Nonetheless, should you already know Categorical, studying Fastify may give you extra choices to make use of in your mission and supply with you a special perspective on internet improvement in Node.js. Its structure actually stands out and that alone makes it value testing. However extra on that later.
Fastify sensible tutorial
For those who assume that Fastify is one thing for you, stick round as a result of we’re going to show how one can implement it in order that it could actually help your personal mission.
Implementation
We’re going to make a easy Fastify mission. The implementation of Fasitfy is sort of just like what you may need skilled with Categorical.js.
Let’s begin with the implementation of fundamental modules: router, app, and server.
To do that, we’re going to recreate three recordsdata:
- server.js – the principle script of the appliance,
- app.js – the script wherein we create a Fastify Node server occasion,
- router.js – Fastify router occasion that helps us encapsulate routes and plugins.
We’re going to create a complete of 4 ts recordsdata within the course of: server.ts, app.ts, router.ts, and routing.ts.
For Server.ts, we merely use our createApp operate from app.ts. We begin by defining a port on which the server can be listening. The file additionally features a easy instance of easy methods to use Fastify’s logger.
The app.ts file is the guts of our code. Right here, we create the createApp operate that initializes the Fastify occasion. Right here, we additionally register plugins by way of app.register (the instance proven registers cors, helmet, and swagger). The router can also be registered right here.
The router.ts defines the best way wherein the shopper requests are dealt with by the appliance endpoints. The router gathers routings for all of the options. Within the instance beneath, we register usersRouting within the router. We move the router to app.js. That’s additionally the place we register it.
There’s additionally routing.ts. It handles routing for customers. We use it to outline each endpoint associated to customers. The instance exhibits how one can outline the get endpoint.
Fastify’s plugins
One of the crucial essential modifications Fastify brings is changing middleware with plugins. Fastify actually does have a robust plugin structure. What are these plugins?
A Fastify plugin could be a set of routes, a server decorator, or just about something. Let’s say we have to ship queries to the database from numerous utility modules. Do we have to connect with the database, export the connection, after which import it into any modules we have to use it in? This works however leads to spaghetti code.
That is the place the Fastify plugin system shines essentially the most. It permits us to inject our dependencies right into a Fastify occasion after which use them wherever now we have entry to the occasion. It additionally helps to simply transfer from a monolithic construction to microservices since every service could be a standalone plugin.
The Fastify ecosystem of plugins is ever-growing. There’s in all probability already a plugin on your favourite database or template language and even performance.
Listed here are a few examples:
- cors -> Fastify-cors,
- helmet -> Fastify-helmet,
- express-jwt -> Fastify-jwt,
- multer -> Fastify-multer,
…. and plenty of extra!
Here’s a full record of all core Fastify plugins.
Getting essentially the most out of Fastify
Fastify additionally has a spread of additional options that permit you to do much more.
Validation
Fastify makes use of Ajv below the hood, which lets you outline validation guidelines with JSON schema; Study extra about how validation works in Fastify.
Logging
Fastify makes use of a quick and versatile logger: pino. The logger occasion is obtainable on the Fastify server occasion (corresponding to Fastify.log.data(“…”)) and on all Request objects (corresponding to request.log.data(“…”));
Logger utilization is easy. You simply have to allow it as a result of it’s disabled as default:
Then, you need to use it like this:
Error dealing with
Fastify gives a setErrorHandler() methodology, which lets you explicitly specify a operate for error dealing with. For extra, you may specify completely different Fastify error handlers utilizing plugins.
Fastify tries to catch as many uncaught errors as it could actually with out hindering efficiency. This consists of:
- synchronous routes: app.get(‘/’, () => { throw new Error(‘kaboom’) })
- async routes: app.get(‘/’, async () => { throw new Error(‘kaboom’) })
Decorators
They permit us to customise core Fastify objects such because the server occasion itself and any request and reply objects used through the HTTP request lifecycle. The decorators API can be utilized to connect any kind of property to the core objects, e.g. features, plain objects, or native sorts.
Fastify studying assets
Wish to proceed your analysis into Fastify? There are quite a lot of attention-grabbing assets on the market that may allow you to out:
Official Fastify web site
- Absolutely, you should begin with the official Fastify web site, which has the docs, benchmarks, and extra.
Fastify npm
- The package deal supervisor for Node.js has a Fastify web page with tons of helpful info, together with variations, dependencies, guides, and whatnot.
Fastify’s Github
- The go-to place for the newest contributions to the Fastify framework codebase.
Migrating from Categorical to Fastify
- A really helpful and sensible article for everybody who needs emigrate from Categorical to Fastify in an present mission. It’s a extremely beneficial learn.
Categorical vs Fastify efficiency comparability
- This text has a really attention-grabbing collection of efficiency information for Categorical and Fastify.
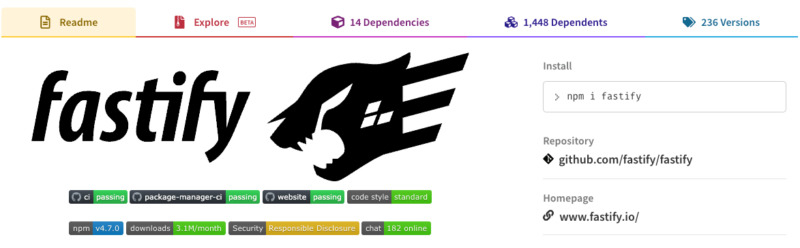
Fastify and internet improvement tendencies
Fastify is continually evolving. The Fastify framework is written in vanilla JavaScript, and as such kind definitions will not be as simple to keep up; nonetheless, since model 2 and past, maintainers and contributors have put in a fantastic effort to enhance the kinds.
Fastify kind system
The kind system was modified in Fastify model 3. The brand new kind system introduces generic constraining and defaulting, plus a brand new solution to outline schema sorts corresponding to a request physique, question string, and extra!
Because the group works on bettering framework and sort definition synergy, generally elements of the API won’t be typed or could also be typed incorrectly. That’s why it’s drastically appropriate with Typescript.
Fastify – conclusions & classes realized
Contemplating simply how a lot builders love Categorical, one would possibly ask – do we actually want yet one more internet framework for Node.js?
My private opinion is a convincing: YES. Wholesome competitors all the time spurs progress, resulting in a unending drive in direction of sooner, higher, and extra environment friendly options.
Fastify actually has the makings of a fantastic framework. I already think about it to be a fantastic selection for each internet improvement and API improvement, for quite a lot of causes:
- It’s quick, economical, and helps TypeScript out-of-the-box. The latter is an enormous bonus for giant industrial tasks.
- It really works nice with a wide range of revolutionary applied sciences frequent in modern-day internet improvement, corresponding to AWS Lambda, or GraphQL.
- It performs to its strengths, specializing in dealing with the assets of your server effectively and quick
Nonetheless, good previous Categorical remains to be right here and is one thing all of us can depend on. On the finish of the day, there’s no good motive to completely restrict your self to 1 framework, and shun all of the newcomers. Alternatively, you shouldn’t all of the sudden go right into a frenzy of refactoring each internet utility to Fastify both.
Within the close to future, it’s secure to say that Fastify won’t overtake Categorical by way of reputation (Categorical with its 25 million downloads is gigantic), however I feel that it’s going to actually develop, and except some new “competitor” is discovered, it could at some point develop into as fashionable as Categorical. It actually feels prefer it has all it takes.
I hope that by the point you begin your subsequent Node.js mission, you’ll have executed sufficient analysis into Fastify to make an knowledgeable selection on whether or not it’s a good match for the event!
Node.js is a key know-how within the improvement of microservices
Study extra concerning the newest microservices tendencies from the State of Microservices report