TypeScript got here round to reinforce JavaScript with numerous options builders had longed for. It made JavaScript much less error-prone and extra scalable by sturdy typing. However the evolution of TypeScript is way from completed. Let’s check out the options added in variations 4.1-4.7 with particular consideration for those who make a distinction in real-life eventualities I encountered in my tasks.
Do you want TypeScript? You most likely already know that, however you’ve obtained firm. Plenty of firm.
There are lots of people who swear by TypeScript’s greatest benefits resembling:
- static typing, which drastically improved the predictability of the code,
- improved IDE expertise, as sorts make it simpler for varied instruments (e.g. VS Code’s Intellisense, or TypeScript’s typically management movement evaluation provided by the kind checker) to supply extremely contextual clever code completion options,
- simpler debugging and higher Developer Expertise as a complete.
Simply how many individuals are we speaking about? Nicely…
TypeScript’s recognition
In accordance with our State of Frontend 2022 report on frontend tendencies, as many as 84.1 % of frontend builders used TypeScript within the final 12 months previous their collaborating within the survey.
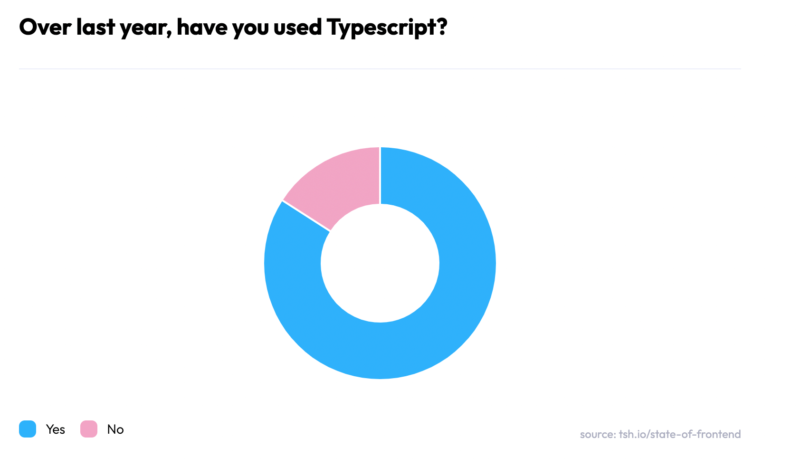
Marcin Gajda, Frontend Staff Supervisor at The Software program Home, agrees that builders universally embraced TypeScript because the go-to method of writing JavaScript code and the development will proceed within the coming years. He believes that the primary purpose for that’s the method TypeScript prevents a complete class of bugs earlier than they’ll even occur, making growth quicker and extra dependable. He provides that:
Certainly, the analysis exhibits that as a lot as 15 % of JavaScript bugs could be prevented by switching to TypeScript alone. The best way TypeScript made it simpler to orient oneself in a big complicated database turned JavaScript right into a extra viable choice for big industrial tasks. It’s one thing our TypeScript growth group can undoubtedly agree on.
However sufficient with that. If you happen to made it this far, you most likely know a factor or two about TypeScript already. Let’s get to the brand new options.
For starters, let’s check out how Microsoft chooses to deal with TypeScript releases.
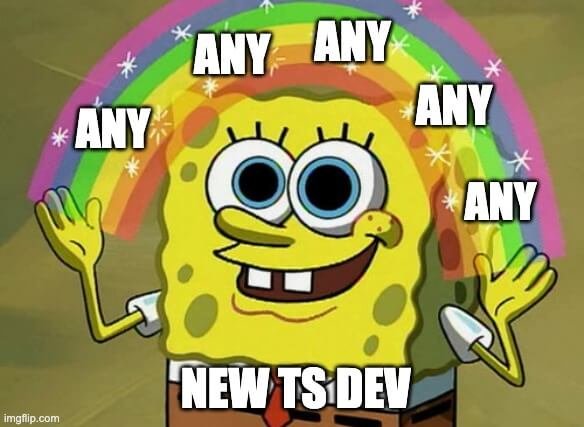
TypeScript releases defined
The discharge cycle for earlier TypeScript variations and the upcoming ones as organized by a group at Microsoft is kind of predictable. Right here is the gist of it:
- There may be a brand new launch each 3 months with little to no variance on this regard.
- Every new launch will get a beta model, adopted by a Launch Candidate model 6 weeks after, adopted by a secure launch within the 2 weeks coming afterward.
- Patches are much less common. The group at Microsoft evaluations the necessity for patches each month and releases them as quickly as they’re prepared.
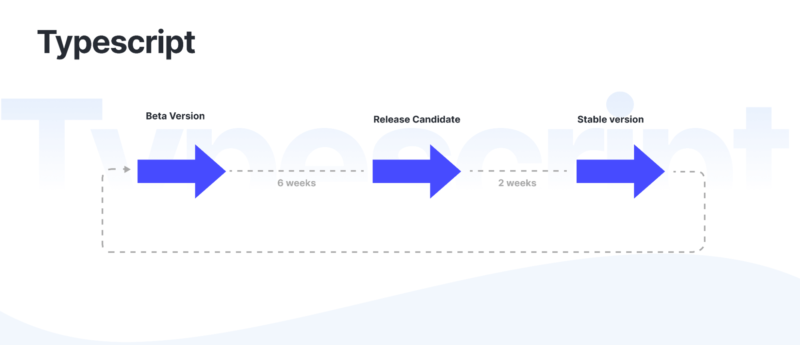
You may learn extra in regards to the concern right here.
TypeScript’s roadmap
With such an orderly launch schedule, it’s fairly simple to foretell the tempo and nature of the language’s progress. A great way to go about that is checking the Roadmap, which incorporates the overview of options and fixes scheduled for a selected launch.
As I’m writing this text, the most recent model we all know something about is TypeScript 4.9, scheduled for launch in November 2022.
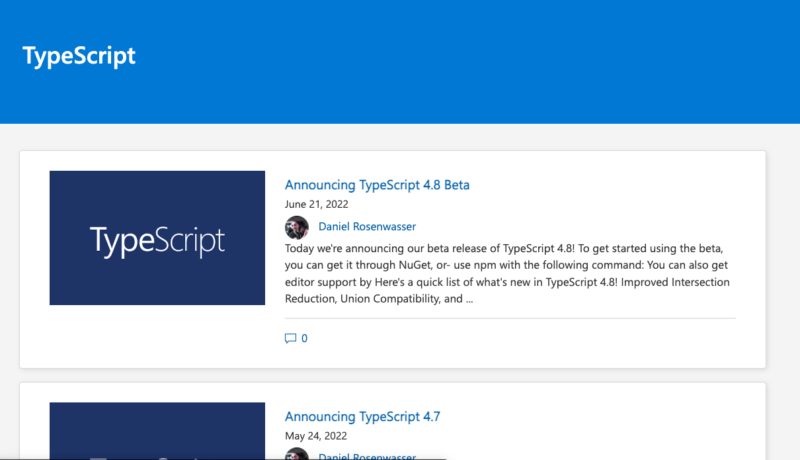
TypeScript model 4 notable options
Ever since TypeScript 4.0 hit the cabinets, the group noticed the discharge of fairly a couple of thrilling options. I’m going to explain intimately the three that I consider to be probably the most related within the on a regular basis work of builders, based mostly on my expertise as a frontend developer at The Software program Home.
Narrowing for variables destructured from Discriminated Unions (model 4.6)
TypeScript has a strong sort inference function. You may typically make some assumptions simply by analyzing code branches. A pleasant real-world instance of the superpower put into use is one thing that I are likely to name: tailored union sorts.
Let’s use a React hook that is ready to question an API for some person names:
I can write a React element that renders an accurate message for the person relying on the present state of the question:
That is good however there’s a JavaScript function that builders like to make use of when coping with objects. It’s referred to as object destructuring. I can use it to shorten the code above like this:
Though this could work in JavaScript with none issues, TypeScript 4.5 would have rested that code on the grounds that it isn’t type-safe. It could not have been satisfied that the knowledge variable within the SUCCESS department is an array.
The rationale for that’s that in TypeScript 4.5, destructured variables misplaced their context.
Because of this, TypeScript has no method of realizing that profitable standing ensures that there’s an object that represents a profitable question state.
Fortunately, this has been improved in TypeScript 4.6 and the above code compiles with none issues. It appears to me that this enchancment may even make builders much less tempted to make use of some TypeScript escape hatches such because the bang operator or type-casting.
Non-obligatory variance annotations (model 4.7)
To grasp this function, there are three ideas you must know slightly about:
- covariance,
- contravariance,
- invariance.
There’s a lot to them. If you would like an in-depth rationalization of them, you’ll be able to undoubtedly discover the data you need on the web. In the present day, I’m going to offer you only a very simplified TLDR model required to proceed with the article.
Let’s say that your app has courses referred to as Person and Admin:
As you’ll be able to see, all admins are customers however not all customers are admins. On this instance, Admin is a subtype of Person. How does this work after I confront these courses with generic typing?
Let’s say that I need to record some customers from the database:
After I anticipate to get customers from the getter, it doesn’t matter if they’re admins or not. On this context, it’s okay to neglect that admins are customers. For example, if I need an electronic mail, it doesn’t matter if I get Admin or Person as a result of each have the e-mail property.
Nevertheless, the issue arises after I anticipate to get an inventory of admins, however all I can do is get an inventory of customers (a few of which aren’t admins). That doesn’t comply as a result of some particular piece of code that makes use of that listAdmins operate could need to verify if an admin is an excellent admin or not. If that operate can’t assure {that a} given person is an admin, it doesn’t match on the kind stage.
The above instance exhibits how the kind T behaves when it’s covariant. In brief phrases, the covariance of a kind T in a generic sort G<T> signifies that G<T> could be subtyped the identical method as T.
Can you discover a counter-example for this? Let’s do that with operate arguments this time:
This time, the result’s totally different.
It’s alright to interchange an admin client with a person client. That’s as a result of if a given operate efficiently consumes customers, it may achieve this with admins by extension because the admin is only a particular sort of person. Nevertheless, a person client can’t get replaced with an admin client. The latter is specialised in working with admins. Some customers could not have the required isSuperAdmin property that could be required right here.
That is additionally an instance of a generic sort that has a contravariant sort. Contravariance occurs when the subtyping route will get flipped within the generic sort context. For example, Admin is a subtype of Person, however within the CollectionConsumer context, it’s the other since CollectionConsumer<Person> is a subtype of CollectionConsumer<Admin>.
TypeScript 4.7 introduces non-compulsory variance annotations into the generic sort definition syntax.
For example, whenever you need to explicitly inform the TypeScript compiler that the generic sort parameter ought to be covariant, you add the out prefix in entrance of the kind. Take into account the next instance:
If you wish to explicitly outline the contravariant sort, you should use the in prefix:
In and out are properly chosen key phrases, as a result of fairly than the educational phrases resembling covariance or contravariance, they signify the place the kind parameter is definitely positioned. The situation could be both within the input of the operate or on the output.
It’s possible you’ll ask: Why ought to I care about that?. TypeScript is basically good at sort inference already and it may spot loads of sort variance issues with none hints.
However, there are no less than two the explanation why this new syntax could be helpful:
- When sorts get actually complicated (e.g. recursive ones). TypeScript’s compiler could lose monitor and infer the kind parameter incorrectly. The trace may help stop that.
- As a result of efficiency issues. Simply good sort inference in TypeScript is nice, it doesn’t imply that it’s quick. You’ll be capable of increase type-checking through the use of in or out key phrases. When the compiler sees them, it may decide sort correctness in variance phrases quicker.
This can be promising for generically-typed libraries. It might increase sort checking in some knowledge validation or type libraries too. In any case, in libraries like these, you anticipate to work on knowledge buildings that you just outline your self.
There may be yet one more case value contemplating: are you able to combine covariance and contravariance collectively. Let’s discover out what occurs whenever you use each in and out key phrases collectively:
It really works, the kind parameter can be utilized in a couple of place in a generic sort. What’s extra, it may be each covariant and contravariant on the similar time. Nevertheless, to fulfill each standards, the kind can’t evolve both right into a subtype or supertype. It must be an actual match. A kind like that is referred to as invariant.
An invariant sort trace could be very helpful. instance is whenever you need to know the precise construction of your type earlier than sending it to an API.
Take into account an interface like this:
Implementation itself shouldn’t be as attention-grabbing as the topic of our evaluation. Let’s assume that there are already some type cases on the kind stage:
The distinction between them is that in the course of the registration of a person, each his first and final identify are required. The person can even enter an affiliation code of one other person that invited them:
However in the course of the replace, solely the primary or final identify could be up to date. On the similar time, solely the outlined fields are to be up to date.
It appears that evidently on a type-level, the registration type could be reused for the updates:
This theoretical API would have an issue with affiliation code throughout updates as further unknown fields typically trigger 400 errors in such circumstances. That’s as a result of RegistrationMutationParameters works as a subtype of ProfileUpdateMutationParameters.
Fortunately, TypeScript 4.7 offers non-compulsory variance annotations you should use to ensure that your type knowledge payload shall be an actual match. The in out prefix is all it’s good to do this:
Now you can set off an error in case the precise match doesn’t happen:
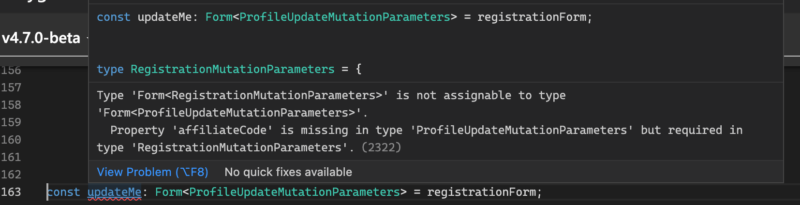
Template Literal Sorts (model 4.1)
This function was an immediate hit the minute builders obtained wind of it. And but, the alternatives it creates proceed to amaze me. However first issues first.
When working with JavaScript recordsdata, there’s a function referred to as Template Literals. It allows you to interpolate dynamic values inside predefined strings:
From TypeScript 4.1, you should use analogous syntax on a type-level:
This will grow to be very useful when used along with generic sorts. Let’s assume you’ve a operate able to including a prefix to all property keys in a given object:
The issue with the kind signature of a operate is that you just lose details about the sorts of authentic properties:
Nevertheless, due to the synergy of generic sorts, mapped sorts, and template literal sorts options, it’s attainable to repair that downside:
There are additionally Intrinsic String Manipulation Sorts that allow you to carry out string operations resembling conversion to decrease or higher case:
Nevertheless, it really is at its strongest when mixed along with conditional sorts and their infer key phrase. That’s as a result of it allows you to parse strings on a type-level. This as an illustration allows you to convert property naming conventions resembling snake_case into camelCase on a type-level. What’s most fun is that it permits folks to parse nearly something, even languages resembling CSS or SQL. Simply check out this experiment with CSS selectors.
Here’s a record of superior issues that individuals implement due to that function. I extremely suggest you give it a strive. The extra examples you see, the better it’s to grasp simply how sensible it’s.
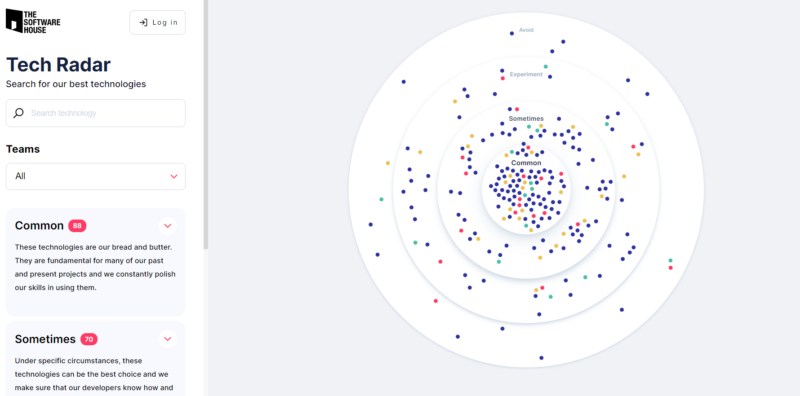
TypeScript 4 variations 4.1 – 4.7 – remaining options overview
In fact, there are loads of different options in TypeScript model 4.1-4.7 that haven’t been described intimately on this article. Many concern points resembling efficiency, enhancements, or the JavaScript/Node.js ecosystem itself.
Since I’m primarily in TypeScript as a programming language, I omitted them right here. The aforementioned DevBlogs useful resource is an effective way to evaluation all of the options. Nonetheless, it’s value it to rapidly record different essential adjustments in TypeScript.
Different TypeScript 4.1-4.7 options to concentrate to
Characteristic | Why care? |
Key remapping | Permits easy manipulation of object keys by offering the “as” key phrase, putting off varied workarounds and straightforward methods. |
Improved sort alias preservation | Due to this out-of-the-box enchancment, sort errors can get extra readable for complicated and longish union sorts that comprise references to outlined sorts. |
Separate write sort properties | Property getters and setters now not must be the identical sort. It creates a chance for accepting varied sorts that may signify knowledge resembling quantity | string | Date whereas preserving a getter, narrowed right down to only a Date. |
Image and Template String Sample Index Signatures | In addition to enabling [index: symbol]: any syntax, you should use indexing via a string sample resembling [optName: `data-${string}`]: any. |
The Awaited Kind and Promise Enhancements | Nails the issue with sorts resembling Promise<Promise<…>> |
Supporting lib from node_modules | TypeScript model upgrades could grow to be much less painful in case of breaking adjustments within the built-in library typings, resembling DOM APIs. In different phrases, you should use the express lib model you’ve put in by npm. |
Management Circulation Evaluation enhancements | This function could prevent from superfluous type-casts in case of if statements that already decide a attainable sort within the particular code department. |
ECMAScript Module Help in Node.js | Since Node.js lastly obtained assist for ESM (ECMAScript modules), TypeScript needed to catch up and introduce a few of the options that make this new Node.js function extra usable from a TypeScript perspective. |
Controle over module detection |
TypeScript tendencies – what does the long run maintain?
What do all of the adjustments imply for the current and way forward for TypeScript?
The sort definition problem
Can sort definitions stay highly effective, whereas additionally avoiding giving devs a steep studying curve? This can be a query that the group at Microsoft must reply.
TypeScript builds on JavaScript and the latter is a really dynamic language. It isn’t a simple job to supply sturdy sort security with out compromising on the flexibility that JavaScript affords. Nonetheless, plainly TypeScript maintainers enhance JavaScript sort security an increasing number of with every new TypeScript launch.
Having stated that, there are builders that don’t like spending an excessive amount of time on sort definitions. They aren’t more likely to profit a lot from all of those fancy language options and enhancements instantly of their work.
In any case, our most important job as builders in industrial tasks is simply to make runtime code fulfill enterprise acceptance standards.
The excellent news for them is that TypeScript’s longish and hard-to-understand sort definition points ought to now not be such an issue. TypeScript’s out-of-the-box error messages are clearly getting higher. That in flip makes it simpler for the authors of third-party libraries to incorporate extra useful error messages.
Developer Expertise would be the precedence
The sort concern clearly exhibits that one in every of TypeScript’s authentic promoting factors was enhancing the expertise of coding in JavaScript. It can proceed to be on the agenda as TypeScript devs attempt to steadiness sophistication with simplicity.
For now, they appear to be succeeding. For instance, a junior developer might not be required to know the way to use Template Literal Sorts magic, however they’ll nonetheless profit from this function not directly through the use of a library resembling kysely. Due to the Typesfeatures, the library can counsel precise desk columns in SQL queries to these much less skilled devs:
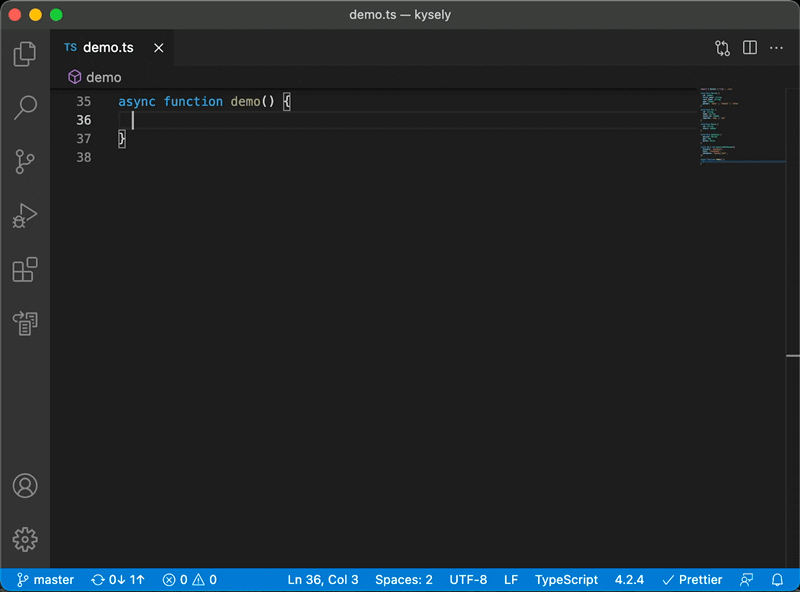
Totally different strokes for various people?
It’s at all times thrilling to see what superior TypeScript customers are capable of obtain when a brand new language function exhibits up within the newest launch.
It appears to me that as TypeScript turns into greater and larger, it’ll more and more have the capability to enchantment to totally different person teams for various causes.
- Juniors will use it as a result of it’s well-liked and that’s what everyone seems to be doing.
- Static-typing seniors will seize the chance to guard juniors from having runtime errors.
With that stated, what sort of new options are you able to anticipate sooner or later?
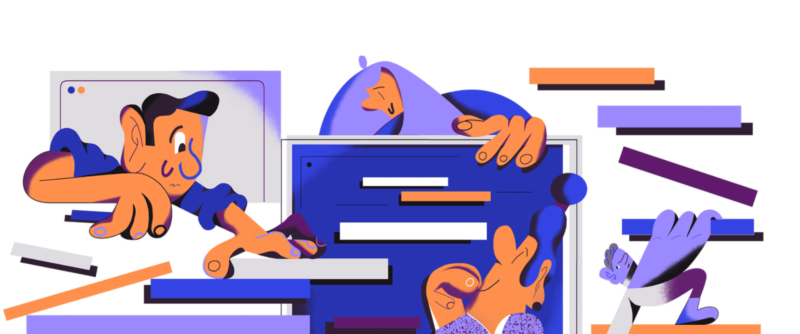
New TypeScript options I’m ready for
There are some issues I want to see in TypeScript. A few of them usually tend to occur, some much less so.
Static error evaluation
As a consequence of TypeScript’s acknowledged purpose of bringing static typing to the JavaScript world in a non-invasive method, TypeScript introduces non-compulsory static typing. Because of this, you at all times have a few escape hatches the place you’ll be able to write unrestricted JavaScript code.
Nevertheless, it appears to me that TypeScript can nonetheless shut some huge gaps for runtime error issues whereas conserving sorts non-compulsory.
I want that TypeScript launched sound static error typing so {that a} dev would know what error they’ll anticipate in that Promise.catch operate callback. However that’s not possible to occur because of some technical limitations. There are additionally some customized workarounds for this, which loads of builders know.
Non-structural sorts
Along with enhancing static evaluation of present JavaScript code, TypeScript can add some worth to it on a kind stage. It’s value remembering that though TypeScript started as a structural sort system, there may be nonetheless an ongoing 8-year debate about extending it to assist non-structural sorts.
What excites me is that this debate lastly obtained into the long run roadmap final yr and receives updates to at the present time. Who is aware of, maybe that is going to be the following large factor in TypeScript?
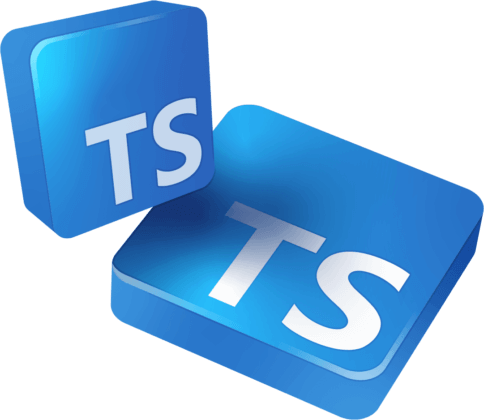
New TypeScript options – key takeaways
It amazes me how TypeScript advanced through the years. It began as a modest static evaluation helper with the purpose to make the overly versatile JavaScript world a bit extra predictable.
Then, it turned a software that detects sort pitfalls in an more and more sensible and seemingly automated method.
Lastly, it matured right into a resolution that helps design higher APIs for issues resembling builders’ favourite ready-to-use libraries.
And it continues to evolve.
My overview of the most recent TypeScript options leaves me with a few ideas:
- Enhancements in discriminated unions are getting us nearer to considerably enhancing a developer’s life with out too large an affect on preferences of code construction.
- Kind inference is getting actually highly effective, particularly full with different options resembling sort guard , sort string, non-compulsory chaining, or recursive sort aliases.
- However on the similar time, thrilling superior options come out rapidly. A few of them could appear nearly tutorial. However then, a library writer comes up with an experimental venture that makes use of that Template Literal Kind in an sudden method, making it out there to a bigger crowd.
In brief, there may be loads of enjoyable available and anticipated from all of you TypeScripts followers on the market!